mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-11 11:16:09 -05:00
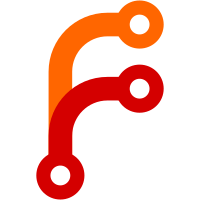
There are no changes to behavior. Changes in this commit are all additions, and are easiest to review using "git diff -U0 --word-diff-regex=." options. Motivation for this change is to keep util functions with really generic names like "Split" and "Join" out of the global namespace so it is easier to see where these functions are defined, and so they don't interfere with function overloading, especially since the util library is a dependency of the kernel library and intended to be used with external code.
101 lines
3.6 KiB
C++
101 lines
3.6 KiB
C++
// Copyright (c) 2012-2022 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <config/bitcoin-config.h> // IWYU pragma: keep
|
|
|
|
#include <clientversion.h>
|
|
#include <util/string.h>
|
|
#include <util/translation.h>
|
|
|
|
#include <tinyformat.h>
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
using util::Join;
|
|
|
|
/**
|
|
* Name of client reported in the 'version' message. Report the same name
|
|
* for both bitcoind and bitcoin-qt, to make it harder for attackers to
|
|
* target servers or GUI users specifically.
|
|
*/
|
|
const std::string CLIENT_NAME("Satoshi");
|
|
|
|
|
|
#ifdef HAVE_BUILD_INFO
|
|
#include <obj/build.h>
|
|
// The <obj/build.h>, which is generated by the build environment (share/genbuild.sh),
|
|
// could contain only one line of the following:
|
|
// - "#define BUILD_GIT_TAG ...", if the top commit is tagged
|
|
// - "#define BUILD_GIT_COMMIT ...", if the top commit is not tagged
|
|
// - "// No build information available", if proper git information is not available
|
|
#endif
|
|
|
|
//! git will put "#define GIT_COMMIT_ID ..." on the next line inside archives. $Format:%n#define GIT_COMMIT_ID "%H"$
|
|
|
|
#ifdef BUILD_GIT_TAG
|
|
#define BUILD_DESC BUILD_GIT_TAG
|
|
#define BUILD_SUFFIX ""
|
|
#else
|
|
#define BUILD_DESC "v" PACKAGE_VERSION
|
|
#if CLIENT_VERSION_IS_RELEASE
|
|
#define BUILD_SUFFIX ""
|
|
#elif defined(BUILD_GIT_COMMIT)
|
|
#define BUILD_SUFFIX "-" BUILD_GIT_COMMIT
|
|
#elif defined(GIT_COMMIT_ID)
|
|
#define BUILD_SUFFIX "-g" GIT_COMMIT_ID
|
|
#else
|
|
#define BUILD_SUFFIX "-unk"
|
|
#endif
|
|
#endif
|
|
|
|
static std::string FormatVersion(int nVersion)
|
|
{
|
|
return strprintf("%d.%d.%d", nVersion / 10000, (nVersion / 100) % 100, nVersion % 100);
|
|
}
|
|
|
|
std::string FormatFullVersion()
|
|
{
|
|
static const std::string CLIENT_BUILD(BUILD_DESC BUILD_SUFFIX);
|
|
return CLIENT_BUILD;
|
|
}
|
|
|
|
/**
|
|
* Format the subversion field according to BIP 14 spec (https://github.com/bitcoin/bips/blob/master/bip-0014.mediawiki)
|
|
*/
|
|
std::string FormatSubVersion(const std::string& name, int nClientVersion, const std::vector<std::string>& comments)
|
|
{
|
|
std::string comments_str;
|
|
if (!comments.empty()) comments_str = strprintf("(%s)", Join(comments, "; "));
|
|
return strprintf("/%s:%s%s/", name, FormatVersion(nClientVersion), comments_str);
|
|
}
|
|
|
|
std::string CopyrightHolders(const std::string& strPrefix)
|
|
{
|
|
const auto copyright_devs = strprintf(_(COPYRIGHT_HOLDERS).translated, COPYRIGHT_HOLDERS_SUBSTITUTION);
|
|
std::string strCopyrightHolders = strPrefix + copyright_devs;
|
|
|
|
// Make sure Bitcoin Core copyright is not removed by accident
|
|
if (copyright_devs.find("Bitcoin Core") == std::string::npos) {
|
|
strCopyrightHolders += "\n" + strPrefix + "The Bitcoin Core developers";
|
|
}
|
|
return strCopyrightHolders;
|
|
}
|
|
|
|
std::string LicenseInfo()
|
|
{
|
|
const std::string URL_SOURCE_CODE = "<https://github.com/bitcoin/bitcoin>";
|
|
|
|
return CopyrightHolders(strprintf(_("Copyright (C) %i-%i").translated, 2009, COPYRIGHT_YEAR) + " ") + "\n" +
|
|
"\n" +
|
|
strprintf(_("Please contribute if you find %s useful. "
|
|
"Visit %s for further information about the software.").translated, PACKAGE_NAME, "<" PACKAGE_URL ">") +
|
|
"\n" +
|
|
strprintf(_("The source code is available from %s.").translated, URL_SOURCE_CODE) +
|
|
"\n" +
|
|
"\n" +
|
|
_("This is experimental software.").translated + "\n" +
|
|
strprintf(_("Distributed under the MIT software license, see the accompanying file %s or %s").translated, "COPYING", "<https://opensource.org/licenses/MIT>") +
|
|
"\n";
|
|
}
|