mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-05 10:17:30 -05:00
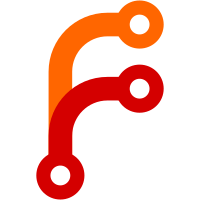
05300c1439
Use SelectionResult in SelectCoins (Andrew Chow)9d9b101d20
Use SelectionResult in AttemptSelection (Andrew Chow)bb50850a44
Use SelectionResult for waste calculation (Andrew Chow)e8f7ae5eb3
Make an OutputGroup for preset inputs (Andrew Chow)51a9c00b4d
Return SelectionResult from SelectCoinsSRD (Andrew Chow)0ef6184575
Return SelectionResult from KnapsackSolver (Andrew Chow)60d2ca72e3
Return SelectionResult from SelectCoinsBnB (Andrew Chow)a339add471
Make member variables of SelectionResult private (Andrew Chow)cbf0b9f4ff
scripted-diff: Use SelectionResult in coin selector tests (Andrew Chow)9d1d86da04
Introduce SelectionResult struct (Andrew Chow)94d851d28c
Fix bnb_search_test to use set equivalence for (Andrew Chow) Pull request description: Instead of returning a set of selected coins and their total value as separate items, encapsulate both of these, and other variables, into a new `SelectionResult` struct. This allows us to have all of the things relevant to a coin selection solution be in a single object. `SelectionResult` enables us to implement the waste calculation in a cleaner way. All of the coin selection functions (`SelectCoinsBnB`, `KnapsackSolver`, `AttemptSelection`, and `SelectCoins`) are changed to use a `SelectionResult` as the output parameter. Based on #22009 ACKs for top commit: laanwj: Code review ACK05300c1439
Tree-SHA512: e4dbb4d78a6cda9c237d230b19e7265591efac5a101a64e6970f0654e2c4f93d13bb5d07b98e8c7b8d37321753dbfc94c28c3a7810cb1c59b5bc29b08a8493ef
105 lines
3.8 KiB
C++
105 lines
3.8 KiB
C++
// Copyright (c) 2012-2020 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <bench/bench.h>
|
|
#include <interfaces/chain.h>
|
|
#include <node/context.h>
|
|
#include <wallet/coinselection.h>
|
|
#include <wallet/spend.h>
|
|
#include <wallet/wallet.h>
|
|
|
|
#include <set>
|
|
|
|
static void addCoin(const CAmount& nValue, const CWallet& wallet, std::vector<std::unique_ptr<CWalletTx>>& wtxs)
|
|
{
|
|
static int nextLockTime = 0;
|
|
CMutableTransaction tx;
|
|
tx.nLockTime = nextLockTime++; // so all transactions get different hashes
|
|
tx.vout.resize(1);
|
|
tx.vout[0].nValue = nValue;
|
|
wtxs.push_back(std::make_unique<CWalletTx>(MakeTransactionRef(std::move(tx)), TxStateInactive{}));
|
|
}
|
|
|
|
// Simple benchmark for wallet coin selection. Note that it maybe be necessary
|
|
// to build up more complicated scenarios in order to get meaningful
|
|
// measurements of performance. From laanwj, "Wallet coin selection is probably
|
|
// the hardest, as you need a wider selection of scenarios, just testing the
|
|
// same one over and over isn't too useful. Generating random isn't useful
|
|
// either for measurements."
|
|
// (https://github.com/bitcoin/bitcoin/issues/7883#issuecomment-224807484)
|
|
static void CoinSelection(benchmark::Bench& bench)
|
|
{
|
|
NodeContext node;
|
|
auto chain = interfaces::MakeChain(node);
|
|
CWallet wallet(chain.get(), "", gArgs, CreateDummyWalletDatabase());
|
|
std::vector<std::unique_ptr<CWalletTx>> wtxs;
|
|
LOCK(wallet.cs_wallet);
|
|
|
|
// Add coins.
|
|
for (int i = 0; i < 1000; ++i) {
|
|
addCoin(1000 * COIN, wallet, wtxs);
|
|
}
|
|
addCoin(3 * COIN, wallet, wtxs);
|
|
|
|
// Create coins
|
|
std::vector<COutput> coins;
|
|
for (const auto& wtx : wtxs) {
|
|
coins.emplace_back(wallet, *wtx, 0 /* iIn */, 6 * 24 /* nDepthIn */, true /* spendable */, true /* solvable */, true /* safe */);
|
|
}
|
|
|
|
const CoinEligibilityFilter filter_standard(1, 6, 0);
|
|
const CoinSelectionParams coin_selection_params(/* change_output_size= */ 34,
|
|
/* change_spend_size= */ 148, /* effective_feerate= */ CFeeRate(0),
|
|
/* long_term_feerate= */ CFeeRate(0), /* discard_feerate= */ CFeeRate(0),
|
|
/* tx_noinputs_size= */ 0, /* avoid_partial= */ false);
|
|
bench.run([&] {
|
|
auto result = AttemptSelection(wallet, 1003 * COIN, filter_standard, coins, coin_selection_params);
|
|
assert(result);
|
|
assert(result->GetSelectedValue() == 1003 * COIN);
|
|
assert(result->GetInputSet().size() == 2);
|
|
});
|
|
}
|
|
|
|
typedef std::set<CInputCoin> CoinSet;
|
|
|
|
// Copied from src/wallet/test/coinselector_tests.cpp
|
|
static void add_coin(const CAmount& nValue, int nInput, std::vector<OutputGroup>& set)
|
|
{
|
|
CMutableTransaction tx;
|
|
tx.vout.resize(nInput + 1);
|
|
tx.vout[nInput].nValue = nValue;
|
|
CInputCoin coin(MakeTransactionRef(tx), nInput);
|
|
set.emplace_back();
|
|
set.back().Insert(coin, 0, true, 0, 0, false);
|
|
}
|
|
// Copied from src/wallet/test/coinselector_tests.cpp
|
|
static CAmount make_hard_case(int utxos, std::vector<OutputGroup>& utxo_pool)
|
|
{
|
|
utxo_pool.clear();
|
|
CAmount target = 0;
|
|
for (int i = 0; i < utxos; ++i) {
|
|
target += (CAmount)1 << (utxos+i);
|
|
add_coin((CAmount)1 << (utxos+i), 2*i, utxo_pool);
|
|
add_coin(((CAmount)1 << (utxos+i)) + ((CAmount)1 << (utxos-1-i)), 2*i + 1, utxo_pool);
|
|
}
|
|
return target;
|
|
}
|
|
|
|
static void BnBExhaustion(benchmark::Bench& bench)
|
|
{
|
|
// Setup
|
|
std::vector<OutputGroup> utxo_pool;
|
|
|
|
bench.run([&] {
|
|
// Benchmark
|
|
CAmount target = make_hard_case(17, utxo_pool);
|
|
SelectCoinsBnB(utxo_pool, target, 0); // Should exhaust
|
|
|
|
// Cleanup
|
|
utxo_pool.clear();
|
|
});
|
|
}
|
|
|
|
BENCHMARK(CoinSelection);
|
|
BENCHMARK(BnBExhaustion);
|