mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-24 12:41:41 -05:00
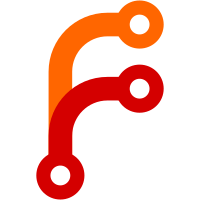
1eac96a503
Compare FromUserHex result against other hex validators and parsers (Lőrinc)19947863e1
Use BOOST_CHECK_EQUAL for optional, arith_uint256, uint256, uint160 (Lőrinc)743ac30e34
Add std::optional support to Boost's equality check (Lőrinc) Pull request description: Enhanced `FromUserHex` coverage by: * Added `std::optional` support to `BOOST_CHECK_EQUAL`, allowing direct comparisons of `std::optional<T>` with other `T` expected values. * Increased fuzz testing for hex parsing to validate against other hex validators and parsers. ---- * Use BOOST_CHECK_EQUAL for https://github.com/bitcoin/bitcoin/pull/30569#discussion_r1706637780 arith_uint256, uint256, uint160 Example error before: > unknown location:0: fatal error: in "validation_chainstatemanager_tests/chainstatemanager_args": std::bad_optional_access: bad_optional_access test/validation_chainstatemanager_tests.cpp:781: last checkpoint after: > test/validation_chainstatemanager_tests.cpp:801: error: in "validation_chainstatemanager_tests/chainstatemanager_args": check set_opts({"-assumevalid=0"}).assumed_valid_block == uint256::ZERO has failed [std::nullopt != 0000000000000000000000000000000000000000000000000000000000000000] ACKs for top commit: stickies-v: re-ACK1eac96a503
ryanofsky: Code review ACK1eac96a503
. Only changes since last review were auto type and fuzz test tweaks. hodlinator: ACK1eac96a503
Tree-SHA512: f1d2c65f0ee4e97830700be5b330189207b11ed0c89a8cebf0f97d43308402a6b3732e10130c79a0c044f7d2eeabfb5359990825aadf02c4ec19428dcd982b00
54 lines
1.9 KiB
C++
54 lines
1.9 KiB
C++
// Copyright (c) 2019-2021 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <core_io.h>
|
|
#include <primitives/block.h>
|
|
#include <pubkey.h>
|
|
#include <rpc/util.h>
|
|
#include <test/fuzz/fuzz.h>
|
|
#include <uint256.h>
|
|
#include <univalue.h>
|
|
#include <util/strencodings.h>
|
|
#include <util/transaction_identifier.h>
|
|
|
|
#include <algorithm>
|
|
#include <cassert>
|
|
#include <cstdint>
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
FUZZ_TARGET(hex)
|
|
{
|
|
const std::string random_hex_string(buffer.begin(), buffer.end());
|
|
const std::vector<unsigned char> data = ParseHex(random_hex_string);
|
|
const std::vector<std::byte> bytes{ParseHex<std::byte>(random_hex_string)};
|
|
assert(std::ranges::equal(AsBytes(Span{data}), bytes));
|
|
const std::string hex_data = HexStr(data);
|
|
if (IsHex(random_hex_string)) {
|
|
assert(ToLower(random_hex_string) == hex_data);
|
|
}
|
|
if (uint256::FromHex(random_hex_string)) {
|
|
assert(random_hex_string.length() == 64);
|
|
assert(Txid::FromHex(random_hex_string));
|
|
assert(Wtxid::FromHex(random_hex_string));
|
|
assert(uint256::FromUserHex(random_hex_string));
|
|
}
|
|
if (const auto result{uint256::FromUserHex(random_hex_string)}) {
|
|
const auto result_string{result->ToString()}; // ToString() returns a fixed-length string without "0x" prefix
|
|
assert(result_string.length() == 64);
|
|
assert(IsHex(result_string));
|
|
assert(TryParseHex(result_string));
|
|
assert(Txid::FromHex(result_string));
|
|
assert(Wtxid::FromHex(result_string));
|
|
assert(uint256::FromHex(result_string));
|
|
}
|
|
try {
|
|
(void)HexToPubKey(random_hex_string);
|
|
} catch (const UniValue&) {
|
|
}
|
|
CBlockHeader block_header;
|
|
(void)DecodeHexBlockHeader(block_header, random_hex_string);
|
|
CBlock block;
|
|
(void)DecodeHexBlk(block, random_hex_string);
|
|
}
|