mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-05 10:17:30 -05:00
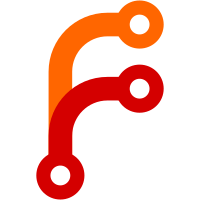
After refactoring general-purpose PSBT and transaction code out of RPC code, for use in the GUI, it's no longer appropriate to throw exceptions. Instead we now return bools for success, and take an output parameter for an error object. We still use JSONRPCError() for the error objects, since only RPC callers actually care about the error codes.
62 lines
2.5 KiB
C++
62 lines
2.5 KiB
C++
// Copyright (c) 2009-2018 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <wallet/psbtwallet.h>
|
|
|
|
bool FillPSBT(const CWallet* pwallet, PartiallySignedTransaction& psbtx, TransactionError& error, bool& complete, int sighash_type, bool sign, bool bip32derivs)
|
|
{
|
|
LOCK(pwallet->cs_wallet);
|
|
// Get all of the previous transactions
|
|
complete = true;
|
|
for (unsigned int i = 0; i < psbtx.tx->vin.size(); ++i) {
|
|
const CTxIn& txin = psbtx.tx->vin[i];
|
|
PSBTInput& input = psbtx.inputs.at(i);
|
|
|
|
if (PSBTInputSigned(input)) {
|
|
continue;
|
|
}
|
|
|
|
// Verify input looks sane. This will check that we have at most one uxto, witness or non-witness.
|
|
if (!input.IsSane()) {
|
|
error = TransactionError::INVALID_PSBT;
|
|
return false;
|
|
}
|
|
|
|
// If we have no utxo, grab it from the wallet.
|
|
if (!input.non_witness_utxo && input.witness_utxo.IsNull()) {
|
|
const uint256& txhash = txin.prevout.hash;
|
|
const auto it = pwallet->mapWallet.find(txhash);
|
|
if (it != pwallet->mapWallet.end()) {
|
|
const CWalletTx& wtx = it->second;
|
|
// We only need the non_witness_utxo, which is a superset of the witness_utxo.
|
|
// The signing code will switch to the smaller witness_utxo if this is ok.
|
|
input.non_witness_utxo = wtx.tx;
|
|
}
|
|
}
|
|
|
|
// Get the Sighash type
|
|
if (sign && input.sighash_type > 0 && input.sighash_type != sighash_type) {
|
|
error = TransactionError::SIGHASH_MISMATCH;
|
|
return false;
|
|
}
|
|
|
|
complete &= SignPSBTInput(HidingSigningProvider(pwallet, !sign, !bip32derivs), psbtx, i, sighash_type);
|
|
}
|
|
|
|
// Fill in the bip32 keypaths and redeemscripts for the outputs so that hardware wallets can identify change
|
|
for (unsigned int i = 0; i < psbtx.tx->vout.size(); ++i) {
|
|
const CTxOut& out = psbtx.tx->vout.at(i);
|
|
PSBTOutput& psbt_out = psbtx.outputs.at(i);
|
|
|
|
// Fill a SignatureData with output info
|
|
SignatureData sigdata;
|
|
psbt_out.FillSignatureData(sigdata);
|
|
|
|
MutableTransactionSignatureCreator creator(psbtx.tx.get_ptr(), 0, out.nValue, 1);
|
|
ProduceSignature(HidingSigningProvider(pwallet, true, !bip32derivs), creator, out.scriptPubKey, sigdata);
|
|
psbt_out.FromSignatureData(sigdata);
|
|
}
|
|
|
|
return true;
|
|
}
|