mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-25 12:51:55 -05:00
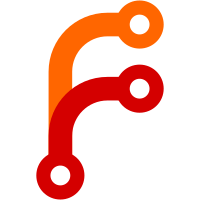
The rpc command verifychain now fails if the dbcache was not sufficient to complete the verification at the specified level and depth. In the same situation, the VerifyDB check during Init will now fail (and lead to an early shutdown) if the user has explicitly specified -checkblocks or -checklevel but the check couldn't be executed because of the limited cache. If the user didn't change any of the two and is using the defaults, log a warning but don't prevent the node from starting up.
68 lines
2.2 KiB
C++
68 lines
2.2 KiB
C++
// Copyright (c) 2021-2022 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_NODE_CHAINSTATE_H
|
|
#define BITCOIN_NODE_CHAINSTATE_H
|
|
|
|
#include <util/translation.h>
|
|
#include <validation.h>
|
|
|
|
#include <cstdint>
|
|
#include <functional>
|
|
#include <tuple>
|
|
|
|
class CTxMemPool;
|
|
|
|
namespace node {
|
|
|
|
struct CacheSizes;
|
|
|
|
struct ChainstateLoadOptions {
|
|
CTxMemPool* mempool{nullptr};
|
|
bool block_tree_db_in_memory{false};
|
|
bool coins_db_in_memory{false};
|
|
bool reindex{false};
|
|
bool reindex_chainstate{false};
|
|
bool prune{false};
|
|
bool require_full_verification{true};
|
|
int64_t check_blocks{DEFAULT_CHECKBLOCKS};
|
|
int64_t check_level{DEFAULT_CHECKLEVEL};
|
|
std::function<bool()> check_interrupt;
|
|
std::function<void()> coins_error_cb;
|
|
};
|
|
|
|
//! Chainstate load status. Simple applications can just check for the success
|
|
//! case, and treat other cases as errors. More complex applications may want to
|
|
//! try reindexing in the generic failure case, and pass an interrupt callback
|
|
//! and exit cleanly in the interrupted case.
|
|
enum class ChainstateLoadStatus {
|
|
SUCCESS,
|
|
FAILURE,
|
|
FAILURE_INCOMPATIBLE_DB,
|
|
FAILURE_INSUFFICIENT_DBCACHE,
|
|
INTERRUPTED,
|
|
};
|
|
|
|
//! Chainstate load status code and optional error string.
|
|
using ChainstateLoadResult = std::tuple<ChainstateLoadStatus, bilingual_str>;
|
|
|
|
/** This sequence can have 4 types of outcomes:
|
|
*
|
|
* 1. Success
|
|
* 2. Shutdown requested
|
|
* - nothing failed but a shutdown was triggered in the middle of the
|
|
* sequence
|
|
* 3. Soft failure
|
|
* - a failure that might be recovered from with a reindex
|
|
* 4. Hard failure
|
|
* - a failure that definitively cannot be recovered from with a reindex
|
|
*
|
|
* LoadChainstate returns a (status code, error string) tuple.
|
|
*/
|
|
ChainstateLoadResult LoadChainstate(ChainstateManager& chainman, const CacheSizes& cache_sizes,
|
|
const ChainstateLoadOptions& options);
|
|
ChainstateLoadResult VerifyLoadedChainstate(ChainstateManager& chainman, const ChainstateLoadOptions& options);
|
|
} // namespace node
|
|
|
|
#endif // BITCOIN_NODE_CHAINSTATE_H
|