mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-03 09:56:38 -05:00
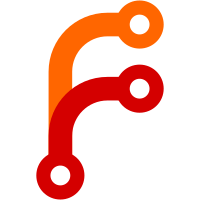
This change drops the last kernel dependency on shutdown.cpp. It also adds new hooks for libbitcoinkernel applications to be able to interrupt kernel operations when the chain tip changes. This is a refactoring that does not affect behavior. (Looking at the code it can appear like the new break statement in the ActivateBestChain function is a change in behavior, but actually the previous StartShutdown call was indirectly triggering a break before, because it was causing m_chainman.m_interrupt to be true. The new code just makes the break more obvious.)
52 lines
1.7 KiB
C++
52 lines
1.7 KiB
C++
// Copyright (c) 2022 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_KERNEL_CHAINSTATEMANAGER_OPTS_H
|
|
#define BITCOIN_KERNEL_CHAINSTATEMANAGER_OPTS_H
|
|
|
|
#include <kernel/notifications_interface.h>
|
|
|
|
#include <arith_uint256.h>
|
|
#include <dbwrapper.h>
|
|
#include <txdb.h>
|
|
#include <uint256.h>
|
|
#include <util/time.h>
|
|
|
|
#include <cstdint>
|
|
#include <functional>
|
|
#include <optional>
|
|
|
|
class CChainParams;
|
|
|
|
static constexpr bool DEFAULT_CHECKPOINTS_ENABLED{true};
|
|
static constexpr auto DEFAULT_MAX_TIP_AGE{24h};
|
|
|
|
namespace kernel {
|
|
|
|
/**
|
|
* An options struct for `ChainstateManager`, more ergonomically referred to as
|
|
* `ChainstateManager::Options` due to the using-declaration in
|
|
* `ChainstateManager`.
|
|
*/
|
|
struct ChainstateManagerOpts {
|
|
const CChainParams& chainparams;
|
|
fs::path datadir;
|
|
const std::function<NodeClock::time_point()> adjusted_time_callback{nullptr};
|
|
std::optional<bool> check_block_index{};
|
|
bool checkpoints_enabled{DEFAULT_CHECKPOINTS_ENABLED};
|
|
//! If set, it will override the minimum work we will assume exists on some valid chain.
|
|
std::optional<arith_uint256> minimum_chain_work{};
|
|
//! If set, it will override the block hash whose ancestors we will assume to have valid scripts without checking them.
|
|
std::optional<uint256> assumed_valid_block{};
|
|
//! If the tip is older than this, the node is considered to be in initial block download.
|
|
std::chrono::seconds max_tip_age{DEFAULT_MAX_TIP_AGE};
|
|
DBOptions block_tree_db{};
|
|
DBOptions coins_db{};
|
|
CoinsViewOptions coins_view{};
|
|
Notifications& notifications;
|
|
};
|
|
|
|
} // namespace kernel
|
|
|
|
#endif // BITCOIN_KERNEL_CHAINSTATEMANAGER_OPTS_H
|