mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-14 11:26:09 -05:00
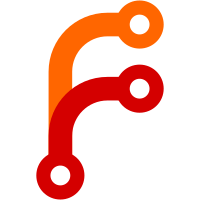
-BEGIN VERIFY SCRIPT- ./contrib/devtools/copyright_header.py update ./ -END VERIFY SCRIPT-
50 lines
1.4 KiB
C++
50 lines
1.4 KiB
C++
// Copyright (c) 2018-2020 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_QT_TEST_APPTESTS_H
|
|
#define BITCOIN_QT_TEST_APPTESTS_H
|
|
|
|
#include <QObject>
|
|
#include <set>
|
|
#include <string>
|
|
#include <utility>
|
|
|
|
class BitcoinApplication;
|
|
class BitcoinGUI;
|
|
class RPCConsole;
|
|
|
|
class AppTests : public QObject
|
|
{
|
|
Q_OBJECT
|
|
public:
|
|
explicit AppTests(BitcoinApplication& app) : m_app(app) {}
|
|
|
|
private Q_SLOTS:
|
|
void appTests();
|
|
void guiTests(BitcoinGUI* window);
|
|
void consoleTests(RPCConsole* console);
|
|
|
|
private:
|
|
//! Add expected callback name to list of pending callbacks.
|
|
void expectCallback(std::string callback) { m_callbacks.emplace(std::move(callback)); }
|
|
|
|
//! RAII helper to remove no-longer-pending callback.
|
|
struct HandleCallback
|
|
{
|
|
std::string m_callback;
|
|
AppTests& m_app_tests;
|
|
~HandleCallback();
|
|
};
|
|
|
|
//! Bitcoin application.
|
|
BitcoinApplication& m_app;
|
|
|
|
//! Set of pending callback names. Used to track expected callbacks and shut
|
|
//! down the app after the last callback has been handled and all tests have
|
|
//! either run or thrown exceptions. This could be a simple int counter
|
|
//! instead of a set of names, but the names might be useful for debugging.
|
|
std::multiset<std::string> m_callbacks;
|
|
};
|
|
|
|
#endif // BITCOIN_QT_TEST_APPTESTS_H
|