mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-05 10:17:30 -05:00
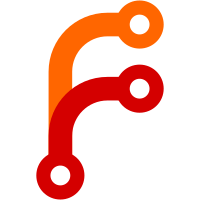
Drop UniValue::getBool method because it is easy to confuse with the UniValue::get_bool method, and could potentially cause bugs. Unlike get_bool, getBool doesn't ensure that the value is a boolean and returns false for all integer, string, array, and object values instead of throwing an exceptions. The getBool method is also redundant because it is an alias for isTrue. There were only 5 getBool() calls in the codebase, so this commit replaces them with isTrue() or get_bool() calls as appropriate. These changes were originally made by MarcoFalke in https://github.com/bitcoin/bitcoin/pull/26213 but were dropped to limit the scope of that PR. Co-authored-by: MarcoFalke <*~=`'#}+{/-|&$^_@721217.xyz>
104 lines
3.6 KiB
C++
104 lines
3.6 KiB
C++
// Copyright (c) 2019-2021 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
//
|
|
#include <test/util/setup_common.h>
|
|
#include <common/run_command.h>
|
|
#include <univalue.h>
|
|
|
|
#ifdef ENABLE_EXTERNAL_SIGNER
|
|
#if defined(__GNUC__)
|
|
// Boost 1.78 requires the following workaround.
|
|
// See: https://github.com/boostorg/process/issues/235
|
|
#pragma GCC diagnostic push
|
|
#pragma GCC diagnostic ignored "-Wnarrowing"
|
|
#endif
|
|
#include <boost/process.hpp>
|
|
#if defined(__GNUC__)
|
|
#pragma GCC diagnostic pop
|
|
#endif
|
|
#endif // ENABLE_EXTERNAL_SIGNER
|
|
|
|
#include <boost/test/unit_test.hpp>
|
|
|
|
BOOST_FIXTURE_TEST_SUITE(system_tests, BasicTestingSetup)
|
|
|
|
// At least one test is required (in case ENABLE_EXTERNAL_SIGNER is not defined).
|
|
// Workaround for https://github.com/bitcoin/bitcoin/issues/19128
|
|
BOOST_AUTO_TEST_CASE(dummy)
|
|
{
|
|
BOOST_CHECK(true);
|
|
}
|
|
|
|
#ifdef ENABLE_EXTERNAL_SIGNER
|
|
|
|
BOOST_AUTO_TEST_CASE(run_command)
|
|
{
|
|
{
|
|
const UniValue result = RunCommandParseJSON("");
|
|
BOOST_CHECK(result.isNull());
|
|
}
|
|
{
|
|
#ifdef WIN32
|
|
const UniValue result = RunCommandParseJSON("cmd.exe /c echo {\"success\": true}");
|
|
#else
|
|
const UniValue result = RunCommandParseJSON("echo \"{\"success\": true}\"");
|
|
#endif
|
|
BOOST_CHECK(result.isObject());
|
|
const UniValue& success = find_value(result, "success");
|
|
BOOST_CHECK(!success.isNull());
|
|
BOOST_CHECK_EQUAL(success.get_bool(), true);
|
|
}
|
|
{
|
|
// An invalid command is handled by Boost
|
|
BOOST_CHECK_EXCEPTION(RunCommandParseJSON("invalid_command"), boost::process::process_error, [&](const boost::process::process_error& e) {
|
|
BOOST_CHECK(std::string(e.what()).find("RunCommandParseJSON error:") == std::string::npos);
|
|
BOOST_CHECK_EQUAL(e.code().value(), 2);
|
|
return true;
|
|
});
|
|
}
|
|
{
|
|
// Return non-zero exit code, no output to stderr
|
|
#ifdef WIN32
|
|
const std::string command{"cmd.exe /c call"};
|
|
#else
|
|
const std::string command{"false"};
|
|
#endif
|
|
BOOST_CHECK_EXCEPTION(RunCommandParseJSON(command), std::runtime_error, [&](const std::runtime_error& e) {
|
|
BOOST_CHECK(std::string(e.what()).find(strprintf("RunCommandParseJSON error: process(%s) returned 1: \n", command)) != std::string::npos);
|
|
return true;
|
|
});
|
|
}
|
|
{
|
|
// Return non-zero exit code, with error message for stderr
|
|
#ifdef WIN32
|
|
const std::string command{"cmd.exe /c dir nosuchfile"};
|
|
const std::string expected{"File Not Found"};
|
|
#else
|
|
const std::string command{"ls nosuchfile"};
|
|
const std::string expected{"No such file or directory"};
|
|
#endif
|
|
BOOST_CHECK_EXCEPTION(RunCommandParseJSON(command), std::runtime_error, [&](const std::runtime_error& e) {
|
|
const std::string what(e.what());
|
|
BOOST_CHECK(what.find(strprintf("RunCommandParseJSON error: process(%s) returned", command)) != std::string::npos);
|
|
BOOST_CHECK(what.find(expected) != std::string::npos);
|
|
return true;
|
|
});
|
|
}
|
|
{
|
|
BOOST_REQUIRE_THROW(RunCommandParseJSON("echo \"{\""), std::runtime_error); // Unable to parse JSON
|
|
}
|
|
// Test std::in, except for Windows
|
|
#ifndef WIN32
|
|
{
|
|
const UniValue result = RunCommandParseJSON("cat", "{\"success\": true}");
|
|
BOOST_CHECK(result.isObject());
|
|
const UniValue& success = find_value(result, "success");
|
|
BOOST_CHECK(!success.isNull());
|
|
BOOST_CHECK_EQUAL(success.get_bool(), true);
|
|
}
|
|
#endif
|
|
}
|
|
#endif // ENABLE_EXTERNAL_SIGNER
|
|
|
|
BOOST_AUTO_TEST_SUITE_END()
|