mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-22 12:23:34 -05:00
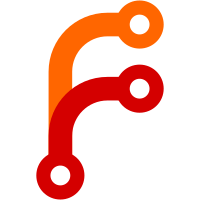
This is an extraction of ArgsManager related functions from util/system into their own common file. Config file related functions are moved to common/config.cpp. The background of this commit is an ongoing effort to decouple the libbitcoinkernel library from the ArgsManager. The ArgsManager belongs into the common library, since the kernel library should not depend on it. See doc/design/libraries.md for more information on this rationale.
116 lines
4.3 KiB
C++
116 lines
4.3 KiB
C++
// Copyright (c) 2020-2022 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <banman.h>
|
|
#include <common/args.h>
|
|
#include <netaddress.h>
|
|
#include <test/fuzz/FuzzedDataProvider.h>
|
|
#include <test/fuzz/fuzz.h>
|
|
#include <test/fuzz/util.h>
|
|
#include <test/fuzz/util/net.h>
|
|
#include <test/util/setup_common.h>
|
|
#include <util/fs.h>
|
|
#include <util/readwritefile.h>
|
|
|
|
#include <cassert>
|
|
#include <cstdint>
|
|
#include <limits>
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
namespace {
|
|
int64_t ConsumeBanTimeOffset(FuzzedDataProvider& fuzzed_data_provider) noexcept
|
|
{
|
|
// Avoid signed integer overflow by capping to int32_t max:
|
|
// banman.cpp:137:73: runtime error: signed integer overflow: 1591700817 + 9223372036854775807 cannot be represented in type 'long'
|
|
return fuzzed_data_provider.ConsumeIntegralInRange<int64_t>(std::numeric_limits<int64_t>::min(), std::numeric_limits<int32_t>::max());
|
|
}
|
|
} // namespace
|
|
|
|
void initialize_banman()
|
|
{
|
|
static const auto testing_setup = MakeNoLogFileContext<>();
|
|
}
|
|
|
|
static bool operator==(const CBanEntry& lhs, const CBanEntry& rhs)
|
|
{
|
|
return lhs.nVersion == rhs.nVersion &&
|
|
lhs.nCreateTime == rhs.nCreateTime &&
|
|
lhs.nBanUntil == rhs.nBanUntil;
|
|
}
|
|
|
|
FUZZ_TARGET_INIT(banman, initialize_banman)
|
|
{
|
|
FuzzedDataProvider fuzzed_data_provider{buffer.data(), buffer.size()};
|
|
SetMockTime(ConsumeTime(fuzzed_data_provider));
|
|
fs::path banlist_file = gArgs.GetDataDirNet() / "fuzzed_banlist";
|
|
|
|
const bool start_with_corrupted_banlist{fuzzed_data_provider.ConsumeBool()};
|
|
bool force_read_and_write_to_err{false};
|
|
if (start_with_corrupted_banlist) {
|
|
assert(WriteBinaryFile(banlist_file + ".json",
|
|
fuzzed_data_provider.ConsumeRandomLengthString()));
|
|
} else {
|
|
force_read_and_write_to_err = fuzzed_data_provider.ConsumeBool();
|
|
if (force_read_and_write_to_err) {
|
|
banlist_file = fs::path{"path"} / "to" / "inaccessible" / "fuzzed_banlist";
|
|
}
|
|
}
|
|
|
|
{
|
|
BanMan ban_man{banlist_file, /*client_interface=*/nullptr, /*default_ban_time=*/ConsumeBanTimeOffset(fuzzed_data_provider)};
|
|
// The complexity is O(N^2), where N is the input size, because each call
|
|
// might call DumpBanlist (or other methods that are at least linear
|
|
// complexity of the input size).
|
|
LIMITED_WHILE(fuzzed_data_provider.ConsumeBool(), 300)
|
|
{
|
|
CallOneOf(
|
|
fuzzed_data_provider,
|
|
[&] {
|
|
ban_man.Ban(ConsumeNetAddr(fuzzed_data_provider),
|
|
ConsumeBanTimeOffset(fuzzed_data_provider), fuzzed_data_provider.ConsumeBool());
|
|
},
|
|
[&] {
|
|
ban_man.Ban(ConsumeSubNet(fuzzed_data_provider),
|
|
ConsumeBanTimeOffset(fuzzed_data_provider), fuzzed_data_provider.ConsumeBool());
|
|
},
|
|
[&] {
|
|
ban_man.ClearBanned();
|
|
},
|
|
[&] {
|
|
ban_man.IsBanned(ConsumeNetAddr(fuzzed_data_provider));
|
|
},
|
|
[&] {
|
|
ban_man.IsBanned(ConsumeSubNet(fuzzed_data_provider));
|
|
},
|
|
[&] {
|
|
ban_man.Unban(ConsumeNetAddr(fuzzed_data_provider));
|
|
},
|
|
[&] {
|
|
ban_man.Unban(ConsumeSubNet(fuzzed_data_provider));
|
|
},
|
|
[&] {
|
|
banmap_t banmap;
|
|
ban_man.GetBanned(banmap);
|
|
},
|
|
[&] {
|
|
ban_man.DumpBanlist();
|
|
},
|
|
[&] {
|
|
ban_man.Discourage(ConsumeNetAddr(fuzzed_data_provider));
|
|
});
|
|
}
|
|
if (!force_read_and_write_to_err) {
|
|
ban_man.DumpBanlist();
|
|
SetMockTime(ConsumeTime(fuzzed_data_provider));
|
|
banmap_t banmap;
|
|
ban_man.GetBanned(banmap);
|
|
BanMan ban_man_read{banlist_file, /*client_interface=*/nullptr, /*default_ban_time=*/0};
|
|
banmap_t banmap_read;
|
|
ban_man_read.GetBanned(banmap_read);
|
|
assert(banmap == banmap_read);
|
|
}
|
|
}
|
|
fs::remove(fs::PathToString(banlist_file + ".json"));
|
|
}
|