mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-04 10:07:27 -05:00
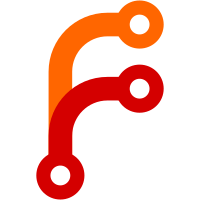
3e9d0bea8d
build: only run high priority benchmarks in 'make check' (furszy)466b54bd4a
bench: surround main() execution with try/catch (furszy)3da7cd2a76
bench: explicitly make all current benchmarks "high" priority (furszy)05b8c76232
bench: add "priority level" to the benchmark framework (furszy)f1593780b8
bench: place benchmark implementation inside benchmark namespace (furszy) Pull request description: This is from today's meeting, a simple "priority level" for the benchmark framework. Will allow us to run certain benchmarks while skip non-prioritized ones in `make check`. By default, `bench_bitcoin` will run all the benchmarks. `make check`will only run the high priority ones, and have marked all the existent benchmarks as "high priority" to retain the current behavior. Could test it by modifying any benchmark priority to something different from "high", and run `bench_bitcoin -priority-level=high` and/or `bench_bitcoin -priority-level=medium,low` (the first command will skip the modified bench while the second one will include it). Note: the second commit could be avoided by having a default arg value for the priority level but.. an explicit set in every `BENCHMARK` macro call makes it less error-prone. ACKs for top commit: kouloumos: re-ACK3e9d0bea8d
achow101: ACK3e9d0bea8d
theStack: re-ACK3e9d0bea8d
stickies-v: re-ACK3e9d0bea8d
Tree-SHA512: ece59bf424c5fc1db335f84caa507476fb8ad8c6151880f1f8289562e17023aae5b5e7de03e8cbba6337bf09215f9be331e9ef51c791c43bce43f7446813b054
36 lines
2 KiB
C++
36 lines
2 KiB
C++
// Copyright (c) 2019 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <bench/bench.h>
|
|
#include <key.h>
|
|
#include <pubkey.h>
|
|
#include <script/descriptor.h>
|
|
#include <script/standard.h>
|
|
|
|
#include <string>
|
|
#include <utility>
|
|
|
|
static void ExpandDescriptor(benchmark::Bench& bench)
|
|
{
|
|
const ECCVerifyHandle verify_handle;
|
|
ECC_Start();
|
|
|
|
const auto desc_str = "sh(wsh(multi(16,03669b8afcec803a0d323e9a17f3ea8e68e8abe5a278020a929adbec52421adbd0,0260b2003c386519fc9eadf2b5cf124dd8eea4c4e68d5e154050a9346ea98ce600,0362a74e399c39ed5593852a30147f2959b56bb827dfa3e60e464b02ccf87dc5e8,0261345b53de74a4d721ef877c255429961b7e43714171ac06168d7e08c542a8b8,02da72e8b46901a65d4374fe6315538d8f368557dda3a1dcf9ea903f3afe7314c8,0318c82dd0b53fd3a932d16e0ba9e278fcc937c582d5781be626ff16e201f72286,0297ccef1ef99f9d73dec9ad37476ddb232f1238aff877af19e72ba04493361009,02e502cfd5c3f972fe9a3e2a18827820638f96b6f347e54d63deb839011fd5765d,03e687710f0e3ebe81c1037074da939d409c0025f17eb86adb9427d28f0f7ae0e9,02c04d3a5274952acdbc76987f3184b346a483d43be40874624b29e3692c1df5af,02ed06e0f418b5b43a7ec01d1d7d27290fa15f75771cb69b642a51471c29c84acd,036d46073cbb9ffee90473f3da429abc8de7f8751199da44485682a989a4bebb24,02f5d1ff7c9029a80a4e36b9a5497027ef7f3e73384a4a94fbfe7c4e9164eec8bc,02e41deffd1b7cce11cde209a781adcffdabd1b91c0ba0375857a2bfd9302419f3,02d76625f7956a7fc505ab02556c23ee72d832f1bac391bcd2d3abce5710a13d06,0399eb0a5487515802dc14544cf10b3666623762fbed2ec38a3975716e2c29c232)))";
|
|
const std::pair<int64_t, int64_t> range = {0, 1000};
|
|
FlatSigningProvider provider;
|
|
std::string error;
|
|
auto desc = Parse(desc_str, provider, error);
|
|
|
|
bench.run([&] {
|
|
for (int i = range.first; i <= range.second; ++i) {
|
|
std::vector<CScript> scripts;
|
|
bool success = desc->Expand(i, provider, scripts, provider);
|
|
assert(success);
|
|
}
|
|
});
|
|
|
|
ECC_Stop();
|
|
}
|
|
|
|
BENCHMARK(ExpandDescriptor, benchmark::PriorityLevel::HIGH);
|