mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-04 10:07:27 -05:00
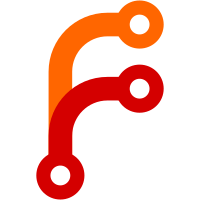
Since the kernel library no longer depends on the system file, move it to the common library instead in accordance to the diagram in doc/design/libraries.md.
76 lines
2.5 KiB
C++
76 lines
2.5 KiB
C++
// Copyright (c) 2018-2022 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <common/args.h>
|
|
#include <common/system.h>
|
|
#include <external_signer.h>
|
|
#include <rpc/protocol.h>
|
|
#include <rpc/server.h>
|
|
#include <rpc/util.h>
|
|
#include <util/strencodings.h>
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
#ifdef ENABLE_EXTERNAL_SIGNER
|
|
|
|
static RPCHelpMan enumeratesigners()
|
|
{
|
|
return RPCHelpMan{"enumeratesigners",
|
|
"Returns a list of external signers from -signer.",
|
|
{},
|
|
RPCResult{
|
|
RPCResult::Type::OBJ, "", "",
|
|
{
|
|
{RPCResult::Type::ARR, "signers", /*optional=*/false, "",
|
|
{
|
|
{RPCResult::Type::OBJ, "", "",
|
|
{
|
|
{RPCResult::Type::STR_HEX, "fingerprint", "Master key fingerprint"},
|
|
{RPCResult::Type::STR, "name", "Device name"},
|
|
}},
|
|
},
|
|
}
|
|
}
|
|
},
|
|
RPCExamples{
|
|
HelpExampleCli("enumeratesigners", "")
|
|
+ HelpExampleRpc("enumeratesigners", "")
|
|
},
|
|
[&](const RPCHelpMan& self, const JSONRPCRequest& request) -> UniValue
|
|
{
|
|
const std::string command = gArgs.GetArg("-signer", "");
|
|
if (command == "") throw JSONRPCError(RPC_MISC_ERROR, "Error: restart bitcoind with -signer=<cmd>");
|
|
const std::string chain = gArgs.GetChainTypeString();
|
|
UniValue signers_res = UniValue::VARR;
|
|
try {
|
|
std::vector<ExternalSigner> signers;
|
|
ExternalSigner::Enumerate(command, signers, chain);
|
|
for (const ExternalSigner& signer : signers) {
|
|
UniValue signer_res = UniValue::VOBJ;
|
|
signer_res.pushKV("fingerprint", signer.m_fingerprint);
|
|
signer_res.pushKV("name", signer.m_name);
|
|
signers_res.push_back(signer_res);
|
|
}
|
|
} catch (const std::exception& e) {
|
|
throw JSONRPCError(RPC_MISC_ERROR, e.what());
|
|
}
|
|
UniValue result(UniValue::VOBJ);
|
|
result.pushKV("signers", signers_res);
|
|
return result;
|
|
}
|
|
};
|
|
}
|
|
|
|
void RegisterSignerRPCCommands(CRPCTable& t)
|
|
{
|
|
static const CRPCCommand commands[]{
|
|
{"signer", &enumeratesigners},
|
|
};
|
|
for (const auto& c : commands) {
|
|
t.appendCommand(c.name, &c);
|
|
}
|
|
}
|
|
|
|
#endif // ENABLE_EXTERNAL_SIGNER
|