mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-11 11:16:09 -05:00
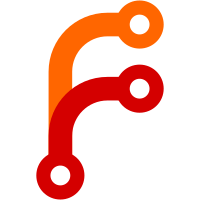
All functions assume that the pointer is never null, so pass by reference, to avoid accidental segfaults at runtime, or at least make them more obvious. Also, remove unused c-style casts in touched lines. Also, add CHECK_NONFATAL checks, to turn segfault crashes into an recoverable runtime error with debug information.
61 lines
1.8 KiB
C++
61 lines
1.8 KiB
C++
// Copyright (c) 2016-2022 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <bench/bench.h>
|
|
#include <bench/data.h>
|
|
|
|
#include <rpc/blockchain.h>
|
|
#include <streams.h>
|
|
#include <test/util/setup_common.h>
|
|
#include <util/chaintype.h>
|
|
#include <validation.h>
|
|
|
|
#include <univalue.h>
|
|
|
|
namespace {
|
|
|
|
struct TestBlockAndIndex {
|
|
const std::unique_ptr<const TestingSetup> testing_setup{MakeNoLogFileContext<const TestingSetup>(ChainType::MAIN)};
|
|
CBlock block{};
|
|
uint256 blockHash{};
|
|
CBlockIndex blockindex{};
|
|
|
|
TestBlockAndIndex()
|
|
{
|
|
DataStream stream{benchmark::data::block413567};
|
|
std::byte a{0};
|
|
stream.write({&a, 1}); // Prevent compaction
|
|
|
|
stream >> TX_WITH_WITNESS(block);
|
|
|
|
blockHash = block.GetHash();
|
|
blockindex.phashBlock = &blockHash;
|
|
blockindex.nBits = 403014710;
|
|
}
|
|
};
|
|
|
|
} // namespace
|
|
|
|
static void BlockToJsonVerbose(benchmark::Bench& bench)
|
|
{
|
|
TestBlockAndIndex data;
|
|
bench.run([&] {
|
|
auto univalue = blockToJSON(data.testing_setup->m_node.chainman->m_blockman, data.block, data.blockindex, data.blockindex, TxVerbosity::SHOW_DETAILS_AND_PREVOUT);
|
|
ankerl::nanobench::doNotOptimizeAway(univalue);
|
|
});
|
|
}
|
|
|
|
BENCHMARK(BlockToJsonVerbose, benchmark::PriorityLevel::HIGH);
|
|
|
|
static void BlockToJsonVerboseWrite(benchmark::Bench& bench)
|
|
{
|
|
TestBlockAndIndex data;
|
|
auto univalue = blockToJSON(data.testing_setup->m_node.chainman->m_blockman, data.block, data.blockindex, data.blockindex, TxVerbosity::SHOW_DETAILS_AND_PREVOUT);
|
|
bench.run([&] {
|
|
auto str = univalue.write();
|
|
ankerl::nanobench::doNotOptimizeAway(str);
|
|
});
|
|
}
|
|
|
|
BENCHMARK(BlockToJsonVerboseWrite, benchmark::PriorityLevel::HIGH);
|