mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-05 10:17:30 -05:00
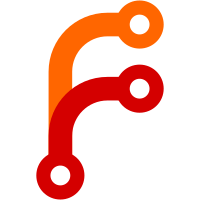
fa32adf9dc
scripted-diff: TxoutType C++11 scoped enum class (MarcoFalke)fa95a694c4
doc: Update outdated txnouttype documentation (MarcoFalke)fa58469c77
rpc: Properly use underlying type in GetAllOutputTypes (MarcoFalke)fa41c65702
rpc: Simplify GetAllOutputTypes with the Join helper (MarcoFalke) Pull request description: Non-scoped enums can accidentally and silently decay into an integral type. Also, the symbol names of the keys are exported to the surrounding (usually global) namespace. Fix both issues by switching to an `enum class TxoutType` in a (mostly) scripted-diff. ACKs for top commit: practicalswift: ACKfa32adf9dc
-- patch looks correct hebasto: re-ACKfa32adf9dc
, since fa5997bd6fc82e16b597ea96e3c5c665f1f174ab (https://github.com/bitcoin/bitcoin/pull/19114#pullrequestreview-421425198) rebased only (verified with `git range-diff`). Tree-SHA512: f42a9db47f9be89fa4bdd8d2fb05a16726286d8b12e3d87327b67d723f91c7d5a57deb4b2ddae9e1d16fee7a5f8c00828b6dc8909c5db680fc5e0a3cf07cd465
236 lines
9 KiB
C++
236 lines
9 KiB
C++
// Copyright (c) 2009-2019 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <core_io.h>
|
|
|
|
#include <consensus/consensus.h>
|
|
#include <consensus/validation.h>
|
|
#include <key_io.h>
|
|
#include <script/script.h>
|
|
#include <script/standard.h>
|
|
#include <serialize.h>
|
|
#include <streams.h>
|
|
#include <univalue.h>
|
|
#include <util/system.h>
|
|
#include <util/strencodings.h>
|
|
|
|
UniValue ValueFromAmount(const CAmount& amount)
|
|
{
|
|
bool sign = amount < 0;
|
|
int64_t n_abs = (sign ? -amount : amount);
|
|
int64_t quotient = n_abs / COIN;
|
|
int64_t remainder = n_abs % COIN;
|
|
return UniValue(UniValue::VNUM,
|
|
strprintf("%s%d.%08d", sign ? "-" : "", quotient, remainder));
|
|
}
|
|
|
|
std::string FormatScript(const CScript& script)
|
|
{
|
|
std::string ret;
|
|
CScript::const_iterator it = script.begin();
|
|
opcodetype op;
|
|
while (it != script.end()) {
|
|
CScript::const_iterator it2 = it;
|
|
std::vector<unsigned char> vch;
|
|
if (script.GetOp(it, op, vch)) {
|
|
if (op == OP_0) {
|
|
ret += "0 ";
|
|
continue;
|
|
} else if ((op >= OP_1 && op <= OP_16) || op == OP_1NEGATE) {
|
|
ret += strprintf("%i ", op - OP_1NEGATE - 1);
|
|
continue;
|
|
} else if (op >= OP_NOP && op <= OP_NOP10) {
|
|
std::string str(GetOpName(op));
|
|
if (str.substr(0, 3) == std::string("OP_")) {
|
|
ret += str.substr(3, std::string::npos) + " ";
|
|
continue;
|
|
}
|
|
}
|
|
if (vch.size() > 0) {
|
|
ret += strprintf("0x%x 0x%x ", HexStr(it2, it - vch.size()), HexStr(it - vch.size(), it));
|
|
} else {
|
|
ret += strprintf("0x%x ", HexStr(it2, it));
|
|
}
|
|
continue;
|
|
}
|
|
ret += strprintf("0x%x ", HexStr(it2, script.end()));
|
|
break;
|
|
}
|
|
return ret.substr(0, ret.size() - 1);
|
|
}
|
|
|
|
const std::map<unsigned char, std::string> mapSigHashTypes = {
|
|
{static_cast<unsigned char>(SIGHASH_ALL), std::string("ALL")},
|
|
{static_cast<unsigned char>(SIGHASH_ALL|SIGHASH_ANYONECANPAY), std::string("ALL|ANYONECANPAY")},
|
|
{static_cast<unsigned char>(SIGHASH_NONE), std::string("NONE")},
|
|
{static_cast<unsigned char>(SIGHASH_NONE|SIGHASH_ANYONECANPAY), std::string("NONE|ANYONECANPAY")},
|
|
{static_cast<unsigned char>(SIGHASH_SINGLE), std::string("SINGLE")},
|
|
{static_cast<unsigned char>(SIGHASH_SINGLE|SIGHASH_ANYONECANPAY), std::string("SINGLE|ANYONECANPAY")},
|
|
};
|
|
|
|
std::string SighashToStr(unsigned char sighash_type)
|
|
{
|
|
const auto& it = mapSigHashTypes.find(sighash_type);
|
|
if (it == mapSigHashTypes.end()) return "";
|
|
return it->second;
|
|
}
|
|
|
|
/**
|
|
* Create the assembly string representation of a CScript object.
|
|
* @param[in] script CScript object to convert into the asm string representation.
|
|
* @param[in] fAttemptSighashDecode Whether to attempt to decode sighash types on data within the script that matches the format
|
|
* of a signature. Only pass true for scripts you believe could contain signatures. For example,
|
|
* pass false, or omit the this argument (defaults to false), for scriptPubKeys.
|
|
*/
|
|
std::string ScriptToAsmStr(const CScript& script, const bool fAttemptSighashDecode)
|
|
{
|
|
std::string str;
|
|
opcodetype opcode;
|
|
std::vector<unsigned char> vch;
|
|
CScript::const_iterator pc = script.begin();
|
|
while (pc < script.end()) {
|
|
if (!str.empty()) {
|
|
str += " ";
|
|
}
|
|
if (!script.GetOp(pc, opcode, vch)) {
|
|
str += "[error]";
|
|
return str;
|
|
}
|
|
if (0 <= opcode && opcode <= OP_PUSHDATA4) {
|
|
if (vch.size() <= static_cast<std::vector<unsigned char>::size_type>(4)) {
|
|
str += strprintf("%d", CScriptNum(vch, false).getint());
|
|
} else {
|
|
// the IsUnspendable check makes sure not to try to decode OP_RETURN data that may match the format of a signature
|
|
if (fAttemptSighashDecode && !script.IsUnspendable()) {
|
|
std::string strSigHashDecode;
|
|
// goal: only attempt to decode a defined sighash type from data that looks like a signature within a scriptSig.
|
|
// this won't decode correctly formatted public keys in Pubkey or Multisig scripts due to
|
|
// the restrictions on the pubkey formats (see IsCompressedOrUncompressedPubKey) being incongruous with the
|
|
// checks in CheckSignatureEncoding.
|
|
if (CheckSignatureEncoding(vch, SCRIPT_VERIFY_STRICTENC, nullptr)) {
|
|
const unsigned char chSigHashType = vch.back();
|
|
if (mapSigHashTypes.count(chSigHashType)) {
|
|
strSigHashDecode = "[" + mapSigHashTypes.find(chSigHashType)->second + "]";
|
|
vch.pop_back(); // remove the sighash type byte. it will be replaced by the decode.
|
|
}
|
|
}
|
|
str += HexStr(vch) + strSigHashDecode;
|
|
} else {
|
|
str += HexStr(vch);
|
|
}
|
|
}
|
|
} else {
|
|
str += GetOpName(opcode);
|
|
}
|
|
}
|
|
return str;
|
|
}
|
|
|
|
std::string EncodeHexTx(const CTransaction& tx, const int serializeFlags)
|
|
{
|
|
CDataStream ssTx(SER_NETWORK, PROTOCOL_VERSION | serializeFlags);
|
|
ssTx << tx;
|
|
return HexStr(ssTx);
|
|
}
|
|
|
|
void ScriptToUniv(const CScript& script, UniValue& out, bool include_address)
|
|
{
|
|
out.pushKV("asm", ScriptToAsmStr(script));
|
|
out.pushKV("hex", HexStr(script));
|
|
|
|
std::vector<std::vector<unsigned char>> solns;
|
|
TxoutType type = Solver(script, solns);
|
|
out.pushKV("type", GetTxnOutputType(type));
|
|
|
|
CTxDestination address;
|
|
if (include_address && ExtractDestination(script, address) && type != TxoutType::PUBKEY) {
|
|
out.pushKV("address", EncodeDestination(address));
|
|
}
|
|
}
|
|
|
|
void ScriptPubKeyToUniv(const CScript& scriptPubKey,
|
|
UniValue& out, bool fIncludeHex)
|
|
{
|
|
TxoutType type;
|
|
std::vector<CTxDestination> addresses;
|
|
int nRequired;
|
|
|
|
out.pushKV("asm", ScriptToAsmStr(scriptPubKey));
|
|
if (fIncludeHex)
|
|
out.pushKV("hex", HexStr(scriptPubKey));
|
|
|
|
if (!ExtractDestinations(scriptPubKey, type, addresses, nRequired) || type == TxoutType::PUBKEY) {
|
|
out.pushKV("type", GetTxnOutputType(type));
|
|
return;
|
|
}
|
|
|
|
out.pushKV("reqSigs", nRequired);
|
|
out.pushKV("type", GetTxnOutputType(type));
|
|
|
|
UniValue a(UniValue::VARR);
|
|
for (const CTxDestination& addr : addresses) {
|
|
a.push_back(EncodeDestination(addr));
|
|
}
|
|
out.pushKV("addresses", a);
|
|
}
|
|
|
|
void TxToUniv(const CTransaction& tx, const uint256& hashBlock, UniValue& entry, bool include_hex, int serialize_flags)
|
|
{
|
|
entry.pushKV("txid", tx.GetHash().GetHex());
|
|
entry.pushKV("hash", tx.GetWitnessHash().GetHex());
|
|
entry.pushKV("version", tx.nVersion);
|
|
entry.pushKV("size", (int)::GetSerializeSize(tx, PROTOCOL_VERSION));
|
|
entry.pushKV("vsize", (GetTransactionWeight(tx) + WITNESS_SCALE_FACTOR - 1) / WITNESS_SCALE_FACTOR);
|
|
entry.pushKV("weight", GetTransactionWeight(tx));
|
|
entry.pushKV("locktime", (int64_t)tx.nLockTime);
|
|
|
|
UniValue vin(UniValue::VARR);
|
|
for (unsigned int i = 0; i < tx.vin.size(); i++) {
|
|
const CTxIn& txin = tx.vin[i];
|
|
UniValue in(UniValue::VOBJ);
|
|
if (tx.IsCoinBase())
|
|
in.pushKV("coinbase", HexStr(txin.scriptSig));
|
|
else {
|
|
in.pushKV("txid", txin.prevout.hash.GetHex());
|
|
in.pushKV("vout", (int64_t)txin.prevout.n);
|
|
UniValue o(UniValue::VOBJ);
|
|
o.pushKV("asm", ScriptToAsmStr(txin.scriptSig, true));
|
|
o.pushKV("hex", HexStr(txin.scriptSig));
|
|
in.pushKV("scriptSig", o);
|
|
}
|
|
if (!tx.vin[i].scriptWitness.IsNull()) {
|
|
UniValue txinwitness(UniValue::VARR);
|
|
for (const auto& item : tx.vin[i].scriptWitness.stack) {
|
|
txinwitness.push_back(HexStr(item));
|
|
}
|
|
in.pushKV("txinwitness", txinwitness);
|
|
}
|
|
in.pushKV("sequence", (int64_t)txin.nSequence);
|
|
vin.push_back(in);
|
|
}
|
|
entry.pushKV("vin", vin);
|
|
|
|
UniValue vout(UniValue::VARR);
|
|
for (unsigned int i = 0; i < tx.vout.size(); i++) {
|
|
const CTxOut& txout = tx.vout[i];
|
|
|
|
UniValue out(UniValue::VOBJ);
|
|
|
|
out.pushKV("value", ValueFromAmount(txout.nValue));
|
|
out.pushKV("n", (int64_t)i);
|
|
|
|
UniValue o(UniValue::VOBJ);
|
|
ScriptPubKeyToUniv(txout.scriptPubKey, o, true);
|
|
out.pushKV("scriptPubKey", o);
|
|
vout.push_back(out);
|
|
}
|
|
entry.pushKV("vout", vout);
|
|
|
|
if (!hashBlock.IsNull())
|
|
entry.pushKV("blockhash", hashBlock.GetHex());
|
|
|
|
if (include_hex) {
|
|
entry.pushKV("hex", EncodeHexTx(tx, serialize_flags)); // The hex-encoded transaction. Used the name "hex" to be consistent with the verbose output of "getrawtransaction".
|
|
}
|
|
}
|