mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-22 12:23:34 -05:00
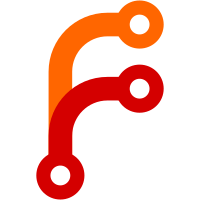
`send(2)` can be interrupted or for another reason it may not fully complete sending all the bytes. We should be ready to retry the send with the remaining bytes. This is what `Sock::SendComplete()` does, thus use it in `Socks5()`. Since `Sock::SendComplete()` takes a `CThreadInterrupt` argument, change also the recv part of `Socks5()` to use `CThreadInterrupt` instead of a boolean. Easier reviewed with `git show -b` (ignore white-space changes).
48 lines
1.8 KiB
C++
48 lines
1.8 KiB
C++
// Copyright (c) 2020-2022 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <netaddress.h>
|
|
#include <netbase.h>
|
|
#include <test/fuzz/FuzzedDataProvider.h>
|
|
#include <test/fuzz/fuzz.h>
|
|
#include <test/fuzz/util.h>
|
|
#include <test/fuzz/util/net.h>
|
|
#include <test/util/setup_common.h>
|
|
|
|
#include <cstdint>
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
extern std::chrono::milliseconds g_socks5_recv_timeout;
|
|
|
|
namespace {
|
|
decltype(g_socks5_recv_timeout) default_socks5_recv_timeout;
|
|
};
|
|
|
|
void initialize_socks5()
|
|
{
|
|
static const auto testing_setup = MakeNoLogFileContext<const BasicTestingSetup>();
|
|
default_socks5_recv_timeout = g_socks5_recv_timeout;
|
|
}
|
|
|
|
FUZZ_TARGET(socks5, .init = initialize_socks5)
|
|
{
|
|
FuzzedDataProvider fuzzed_data_provider{buffer.data(), buffer.size()};
|
|
ProxyCredentials proxy_credentials;
|
|
proxy_credentials.username = fuzzed_data_provider.ConsumeRandomLengthString(512);
|
|
proxy_credentials.password = fuzzed_data_provider.ConsumeRandomLengthString(512);
|
|
if (fuzzed_data_provider.ConsumeBool()) {
|
|
g_socks5_interrupt();
|
|
}
|
|
// Set FUZZED_SOCKET_FAKE_LATENCY=1 to exercise recv timeout code paths. This
|
|
// will slow down fuzzing.
|
|
g_socks5_recv_timeout = (fuzzed_data_provider.ConsumeBool() && std::getenv("FUZZED_SOCKET_FAKE_LATENCY") != nullptr) ? 1ms : default_socks5_recv_timeout;
|
|
FuzzedSock fuzzed_sock = ConsumeSock(fuzzed_data_provider);
|
|
// This Socks5(...) fuzzing harness would have caught CVE-2017-18350 within
|
|
// a few seconds of fuzzing.
|
|
(void)Socks5(fuzzed_data_provider.ConsumeRandomLengthString(512),
|
|
fuzzed_data_provider.ConsumeIntegral<uint16_t>(),
|
|
fuzzed_data_provider.ConsumeBool() ? &proxy_credentials : nullptr,
|
|
fuzzed_sock);
|
|
}
|