mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-21 12:22:50 -05:00
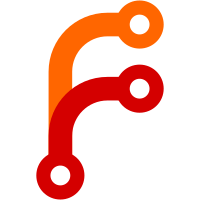
Add common InitConfig function to deduplicate bitcoind and bitcoin-qt code reading config files and creating the datadir. There are a few minor changes in behavior: - In bitcoin-qt, when there is a problem reading the configuration file, the GUI error text has changed from "Error: Cannot parse configuration file:" to "Error reading configuration file:" to be consistent with bitcoind. - In bitcoind, when there is a problem reading the settings.json file, the error text has changed from "Failed loading settings file" to "Settings file could not be read" to be consistent with bitcoin-qt. - In bitcoind, when there is a problem writing the settings.json file, the error text has changed from "Failed saving settings file" to "Settings file could not be written" to be consistent with bitcoin-qt. - In bitcoin-qt, if there datadir is not accessible (e.g. no permission to read), there is an normal error dialog showing "Error: filesystem error: status: Permission denied [.../settings.json]", instead of an uncaught exception
39 lines
1.2 KiB
C++
39 lines
1.2 KiB
C++
// Copyright (c) 2023 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_COMMON_INIT_H
|
|
#define BITCOIN_COMMON_INIT_H
|
|
|
|
#include <util/translation.h>
|
|
|
|
#include <functional>
|
|
#include <optional>
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
class ArgsManager;
|
|
|
|
namespace common {
|
|
enum class ConfigStatus {
|
|
FAILED, //!< Failed generically.
|
|
FAILED_WRITE, //!< Failed to write settings.json
|
|
ABORTED, //!< Aborted by user
|
|
};
|
|
|
|
struct ConfigError {
|
|
ConfigStatus status;
|
|
bilingual_str message{};
|
|
std::vector<std::string> details{};
|
|
};
|
|
|
|
//! Callback function to let the user decide whether to abort loading if
|
|
//! settings.json file exists and can't be parsed, or to ignore the error and
|
|
//! overwrite the file.
|
|
using SettingsAbortFn = std::function<bool(const bilingual_str& message, const std::vector<std::string>& details)>;
|
|
|
|
/* Read config files, and create datadir and settings.json if they don't exist. */
|
|
std::optional<ConfigError> InitConfig(ArgsManager& args, SettingsAbortFn settings_abort_fn = nullptr);
|
|
} // namespace common
|
|
|
|
#endif // BITCOIN_COMMON_INIT_H
|