mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-04 10:07:27 -05:00
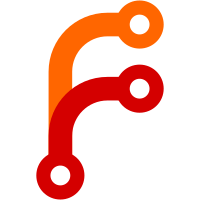
6c7a17a8e0
psbt: support externally provided preimages for Miniscript satisfaction (Antoine Poinsot)840a396029
qa: add a "smart" Miniscript fuzz target (Antoine Poinsot)17e3547241
qa: add a fuzz target generating random nodes from a binary encoding (Antoine Poinsot)611e12502a
qa: functional test Miniscript signing with key and timelocks (Antoine Poinsot)d57b7f2021
refactor: make descriptors in Miniscript functional test more readable (Antoine Poinsot)0a8fc9e200
wallet: check solvability using descriptor in AvailableCoins (Antoine Poinsot)560e62b1e2
script/sign: signing support for Miniscripts with hash preimage challenges (Antoine Poinsot)a2f81b6a8f
script/sign: signing support for Miniscript with timelocks (Antoine Poinsot)61c6d1a844
script/sign: basic signing support for Miniscript descriptors (Antoine Poinsot)4242c1c521
Align 'e' property of or_d and andor with website spec (Pieter Wuille)f5deb41780
Various additional explanations of the satisfaction logic from Pieter (Pieter Wuille)22c5b00345
miniscript: satisfaction support (Antoine Poinsot) Pull request description: This makes the Miniscript descriptors solvable. Note this introduces signing support for much more complex scripts than the wallet was previously able to solve, and the whole tooling isn't provided for a complete Miniscript integration in the wallet. Particularly, the PSBT<->Miniscript integration isn't entirely covered in this PR. ACKs for top commit: achow101: ACK6c7a17a8e0
sipa: utACK6c7a17a8e0
(to the extent that it's not my own code). Tree-SHA512: a71ec002aaf66bd429012caa338fc58384067bcd2f453a46e21d381ed1bacc8e57afb9db57c0fb4bf40de43b30808815e9ebc0ae1fbd9e61df0e7b91a17771cc
226 lines
9 KiB
C++
226 lines
9 KiB
C++
// Copyright (c) 2011-2022 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <key.h>
|
|
#include <policy/policy.h>
|
|
#include <script/interpreter.h>
|
|
#include <script/script.h>
|
|
#include <script/script_error.h>
|
|
#include <script/sign.h>
|
|
#include <script/signingprovider.h>
|
|
#include <test/util/setup_common.h>
|
|
#include <tinyformat.h>
|
|
#include <uint256.h>
|
|
|
|
|
|
#include <boost/test/unit_test.hpp>
|
|
|
|
BOOST_FIXTURE_TEST_SUITE(multisig_tests, BasicTestingSetup)
|
|
|
|
static CScript
|
|
sign_multisig(const CScript& scriptPubKey, const std::vector<CKey>& keys, const CTransaction& transaction, int whichIn)
|
|
{
|
|
uint256 hash = SignatureHash(scriptPubKey, transaction, whichIn, SIGHASH_ALL, 0, SigVersion::BASE);
|
|
|
|
CScript result;
|
|
result << OP_0; // CHECKMULTISIG bug workaround
|
|
for (const CKey &key : keys)
|
|
{
|
|
std::vector<unsigned char> vchSig;
|
|
BOOST_CHECK(key.Sign(hash, vchSig));
|
|
vchSig.push_back((unsigned char)SIGHASH_ALL);
|
|
result << vchSig;
|
|
}
|
|
return result;
|
|
}
|
|
|
|
BOOST_AUTO_TEST_CASE(multisig_verify)
|
|
{
|
|
unsigned int flags = SCRIPT_VERIFY_P2SH | SCRIPT_VERIFY_STRICTENC;
|
|
|
|
ScriptError err;
|
|
CKey key[4];
|
|
CAmount amount = 0;
|
|
for (int i = 0; i < 4; i++)
|
|
key[i].MakeNewKey(true);
|
|
|
|
CScript a_and_b;
|
|
a_and_b << OP_2 << ToByteVector(key[0].GetPubKey()) << ToByteVector(key[1].GetPubKey()) << OP_2 << OP_CHECKMULTISIG;
|
|
|
|
CScript a_or_b;
|
|
a_or_b << OP_1 << ToByteVector(key[0].GetPubKey()) << ToByteVector(key[1].GetPubKey()) << OP_2 << OP_CHECKMULTISIG;
|
|
|
|
CScript escrow;
|
|
escrow << OP_2 << ToByteVector(key[0].GetPubKey()) << ToByteVector(key[1].GetPubKey()) << ToByteVector(key[2].GetPubKey()) << OP_3 << OP_CHECKMULTISIG;
|
|
|
|
CMutableTransaction txFrom; // Funding transaction
|
|
txFrom.vout.resize(3);
|
|
txFrom.vout[0].scriptPubKey = a_and_b;
|
|
txFrom.vout[1].scriptPubKey = a_or_b;
|
|
txFrom.vout[2].scriptPubKey = escrow;
|
|
|
|
CMutableTransaction txTo[3]; // Spending transaction
|
|
for (int i = 0; i < 3; i++)
|
|
{
|
|
txTo[i].vin.resize(1);
|
|
txTo[i].vout.resize(1);
|
|
txTo[i].vin[0].prevout.n = i;
|
|
txTo[i].vin[0].prevout.hash = txFrom.GetHash();
|
|
txTo[i].vout[0].nValue = 1;
|
|
}
|
|
|
|
std::vector<CKey> keys;
|
|
CScript s;
|
|
|
|
// Test a AND b:
|
|
keys.assign(1,key[0]);
|
|
keys.push_back(key[1]);
|
|
s = sign_multisig(a_and_b, keys, CTransaction(txTo[0]), 0);
|
|
BOOST_CHECK(VerifyScript(s, a_and_b, nullptr, flags, MutableTransactionSignatureChecker(&txTo[0], 0, amount, MissingDataBehavior::ASSERT_FAIL), &err));
|
|
BOOST_CHECK_MESSAGE(err == SCRIPT_ERR_OK, ScriptErrorString(err));
|
|
|
|
for (int i = 0; i < 4; i++)
|
|
{
|
|
keys.assign(1,key[i]);
|
|
s = sign_multisig(a_and_b, keys, CTransaction(txTo[0]), 0);
|
|
BOOST_CHECK_MESSAGE(!VerifyScript(s, a_and_b, nullptr, flags, MutableTransactionSignatureChecker(&txTo[0], 0, amount, MissingDataBehavior::ASSERT_FAIL), &err), strprintf("a&b 1: %d", i));
|
|
BOOST_CHECK_MESSAGE(err == SCRIPT_ERR_INVALID_STACK_OPERATION, ScriptErrorString(err));
|
|
|
|
keys.assign(1,key[1]);
|
|
keys.push_back(key[i]);
|
|
s = sign_multisig(a_and_b, keys, CTransaction(txTo[0]), 0);
|
|
BOOST_CHECK_MESSAGE(!VerifyScript(s, a_and_b, nullptr, flags, MutableTransactionSignatureChecker(&txTo[0], 0, amount, MissingDataBehavior::ASSERT_FAIL), &err), strprintf("a&b 2: %d", i));
|
|
BOOST_CHECK_MESSAGE(err == SCRIPT_ERR_EVAL_FALSE, ScriptErrorString(err));
|
|
}
|
|
|
|
// Test a OR b:
|
|
for (int i = 0; i < 4; i++)
|
|
{
|
|
keys.assign(1,key[i]);
|
|
s = sign_multisig(a_or_b, keys, CTransaction(txTo[1]), 0);
|
|
if (i == 0 || i == 1)
|
|
{
|
|
BOOST_CHECK_MESSAGE(VerifyScript(s, a_or_b, nullptr, flags, MutableTransactionSignatureChecker(&txTo[1], 0, amount, MissingDataBehavior::ASSERT_FAIL), &err), strprintf("a|b: %d", i));
|
|
BOOST_CHECK_MESSAGE(err == SCRIPT_ERR_OK, ScriptErrorString(err));
|
|
}
|
|
else
|
|
{
|
|
BOOST_CHECK_MESSAGE(!VerifyScript(s, a_or_b, nullptr, flags, MutableTransactionSignatureChecker(&txTo[1], 0, amount, MissingDataBehavior::ASSERT_FAIL), &err), strprintf("a|b: %d", i));
|
|
BOOST_CHECK_MESSAGE(err == SCRIPT_ERR_EVAL_FALSE, ScriptErrorString(err));
|
|
}
|
|
}
|
|
s.clear();
|
|
s << OP_0 << OP_1;
|
|
BOOST_CHECK(!VerifyScript(s, a_or_b, nullptr, flags, MutableTransactionSignatureChecker(&txTo[1], 0, amount, MissingDataBehavior::ASSERT_FAIL), &err));
|
|
BOOST_CHECK_MESSAGE(err == SCRIPT_ERR_SIG_DER, ScriptErrorString(err));
|
|
|
|
|
|
for (int i = 0; i < 4; i++)
|
|
for (int j = 0; j < 4; j++)
|
|
{
|
|
keys.assign(1,key[i]);
|
|
keys.push_back(key[j]);
|
|
s = sign_multisig(escrow, keys, CTransaction(txTo[2]), 0);
|
|
if (i < j && i < 3 && j < 3)
|
|
{
|
|
BOOST_CHECK_MESSAGE(VerifyScript(s, escrow, nullptr, flags, MutableTransactionSignatureChecker(&txTo[2], 0, amount, MissingDataBehavior::ASSERT_FAIL), &err), strprintf("escrow 1: %d %d", i, j));
|
|
BOOST_CHECK_MESSAGE(err == SCRIPT_ERR_OK, ScriptErrorString(err));
|
|
}
|
|
else
|
|
{
|
|
BOOST_CHECK_MESSAGE(!VerifyScript(s, escrow, nullptr, flags, MutableTransactionSignatureChecker(&txTo[2], 0, amount, MissingDataBehavior::ASSERT_FAIL), &err), strprintf("escrow 2: %d %d", i, j));
|
|
BOOST_CHECK_MESSAGE(err == SCRIPT_ERR_EVAL_FALSE, ScriptErrorString(err));
|
|
}
|
|
}
|
|
}
|
|
|
|
BOOST_AUTO_TEST_CASE(multisig_IsStandard)
|
|
{
|
|
CKey key[4];
|
|
for (int i = 0; i < 4; i++)
|
|
key[i].MakeNewKey(true);
|
|
|
|
const auto is_standard{[](const CScript& spk) {
|
|
TxoutType type;
|
|
bool res{::IsStandard(spk, std::nullopt, type)};
|
|
if (res) {
|
|
BOOST_CHECK_EQUAL(type, TxoutType::MULTISIG);
|
|
}
|
|
return res;
|
|
}};
|
|
|
|
CScript a_and_b;
|
|
a_and_b << OP_2 << ToByteVector(key[0].GetPubKey()) << ToByteVector(key[1].GetPubKey()) << OP_2 << OP_CHECKMULTISIG;
|
|
BOOST_CHECK(is_standard(a_and_b));
|
|
|
|
CScript a_or_b;
|
|
a_or_b << OP_1 << ToByteVector(key[0].GetPubKey()) << ToByteVector(key[1].GetPubKey()) << OP_2 << OP_CHECKMULTISIG;
|
|
BOOST_CHECK(is_standard(a_or_b));
|
|
|
|
CScript escrow;
|
|
escrow << OP_2 << ToByteVector(key[0].GetPubKey()) << ToByteVector(key[1].GetPubKey()) << ToByteVector(key[2].GetPubKey()) << OP_3 << OP_CHECKMULTISIG;
|
|
BOOST_CHECK(is_standard(escrow));
|
|
|
|
CScript one_of_four;
|
|
one_of_four << OP_1 << ToByteVector(key[0].GetPubKey()) << ToByteVector(key[1].GetPubKey()) << ToByteVector(key[2].GetPubKey()) << ToByteVector(key[3].GetPubKey()) << OP_4 << OP_CHECKMULTISIG;
|
|
BOOST_CHECK(!is_standard(one_of_four));
|
|
|
|
CScript malformed[6];
|
|
malformed[0] << OP_3 << ToByteVector(key[0].GetPubKey()) << ToByteVector(key[1].GetPubKey()) << OP_2 << OP_CHECKMULTISIG;
|
|
malformed[1] << OP_2 << ToByteVector(key[0].GetPubKey()) << ToByteVector(key[1].GetPubKey()) << OP_3 << OP_CHECKMULTISIG;
|
|
malformed[2] << OP_0 << ToByteVector(key[0].GetPubKey()) << ToByteVector(key[1].GetPubKey()) << OP_2 << OP_CHECKMULTISIG;
|
|
malformed[3] << OP_1 << ToByteVector(key[0].GetPubKey()) << ToByteVector(key[1].GetPubKey()) << OP_0 << OP_CHECKMULTISIG;
|
|
malformed[4] << OP_1 << ToByteVector(key[0].GetPubKey()) << ToByteVector(key[1].GetPubKey()) << OP_CHECKMULTISIG;
|
|
malformed[5] << OP_1 << ToByteVector(key[0].GetPubKey()) << ToByteVector(key[1].GetPubKey());
|
|
|
|
for (int i = 0; i < 6; i++) {
|
|
BOOST_CHECK(!is_standard(malformed[i]));
|
|
}
|
|
}
|
|
|
|
BOOST_AUTO_TEST_CASE(multisig_Sign)
|
|
{
|
|
// Test SignSignature() (and therefore the version of Solver() that signs transactions)
|
|
FillableSigningProvider keystore;
|
|
CKey key[4];
|
|
for (int i = 0; i < 4; i++)
|
|
{
|
|
key[i].MakeNewKey(true);
|
|
BOOST_CHECK(keystore.AddKey(key[i]));
|
|
}
|
|
|
|
CScript a_and_b;
|
|
a_and_b << OP_2 << ToByteVector(key[0].GetPubKey()) << ToByteVector(key[1].GetPubKey()) << OP_2 << OP_CHECKMULTISIG;
|
|
|
|
CScript a_or_b;
|
|
a_or_b << OP_1 << ToByteVector(key[0].GetPubKey()) << ToByteVector(key[1].GetPubKey()) << OP_2 << OP_CHECKMULTISIG;
|
|
|
|
CScript escrow;
|
|
escrow << OP_2 << ToByteVector(key[0].GetPubKey()) << ToByteVector(key[1].GetPubKey()) << ToByteVector(key[2].GetPubKey()) << OP_3 << OP_CHECKMULTISIG;
|
|
|
|
CMutableTransaction txFrom; // Funding transaction
|
|
txFrom.vout.resize(3);
|
|
txFrom.vout[0].scriptPubKey = a_and_b;
|
|
txFrom.vout[1].scriptPubKey = a_or_b;
|
|
txFrom.vout[2].scriptPubKey = escrow;
|
|
|
|
CMutableTransaction txTo[3]; // Spending transaction
|
|
for (int i = 0; i < 3; i++)
|
|
{
|
|
txTo[i].vin.resize(1);
|
|
txTo[i].vout.resize(1);
|
|
txTo[i].vin[0].prevout.n = i;
|
|
txTo[i].vin[0].prevout.hash = txFrom.GetHash();
|
|
txTo[i].vout[0].nValue = 1;
|
|
}
|
|
|
|
for (int i = 0; i < 3; i++)
|
|
{
|
|
SignatureData empty;
|
|
BOOST_CHECK_MESSAGE(SignSignature(keystore, CTransaction(txFrom), txTo[i], 0, SIGHASH_ALL, empty), strprintf("SignSignature %d", i));
|
|
}
|
|
}
|
|
|
|
|
|
BOOST_AUTO_TEST_SUITE_END()
|