mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-24 12:41:41 -05:00
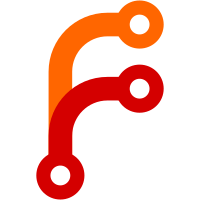
The extra `bilingual_str` argument of the fatal error notifications and `node::AbortNode()` is often unused and when used usually contains the same string as the message argument. It also seems to be confusing, since it is not consistently used for errors requiring user action. For example some assumeutxo fatal errors require the user to do something, but are not translated. So simplify the fatal error and abort node interfaces by only passing a translated string. This slightly changes the fatal errors displayed to the user. Also de-duplicate the abort error log since it is repeated in noui.cpp.
56 lines
1.8 KiB
C++
56 lines
1.8 KiB
C++
// Copyright (c) 2023 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_NODE_KERNEL_NOTIFICATIONS_H
|
|
#define BITCOIN_NODE_KERNEL_NOTIFICATIONS_H
|
|
|
|
#include <kernel/notifications_interface.h>
|
|
|
|
#include <atomic>
|
|
#include <cstdint>
|
|
|
|
class ArgsManager;
|
|
class CBlockIndex;
|
|
enum class SynchronizationState;
|
|
struct bilingual_str;
|
|
|
|
namespace util {
|
|
class SignalInterrupt;
|
|
} // namespace util
|
|
|
|
namespace node {
|
|
|
|
static constexpr int DEFAULT_STOPATHEIGHT{0};
|
|
|
|
class KernelNotifications : public kernel::Notifications
|
|
{
|
|
public:
|
|
KernelNotifications(util::SignalInterrupt& shutdown, std::atomic<int>& exit_status) : m_shutdown(shutdown), m_exit_status{exit_status} {}
|
|
|
|
[[nodiscard]] kernel::InterruptResult blockTip(SynchronizationState state, CBlockIndex& index) override;
|
|
|
|
void headerTip(SynchronizationState state, int64_t height, int64_t timestamp, bool presync) override;
|
|
|
|
void progress(const bilingual_str& title, int progress_percent, bool resume_possible) override;
|
|
|
|
void warning(const bilingual_str& warning) override;
|
|
|
|
void flushError(const bilingual_str& message) override;
|
|
|
|
void fatalError(const bilingual_str& message) override;
|
|
|
|
//! Block height after which blockTip notification will return Interrupted{}, if >0.
|
|
int m_stop_at_height{DEFAULT_STOPATHEIGHT};
|
|
//! Useful for tests, can be set to false to avoid shutdown on fatal error.
|
|
bool m_shutdown_on_fatal_error{true};
|
|
private:
|
|
util::SignalInterrupt& m_shutdown;
|
|
std::atomic<int>& m_exit_status;
|
|
};
|
|
|
|
void ReadNotificationArgs(const ArgsManager& args, KernelNotifications& notifications);
|
|
|
|
} // namespace node
|
|
|
|
#endif // BITCOIN_NODE_KERNEL_NOTIFICATIONS_H
|