mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-05 10:17:30 -05:00
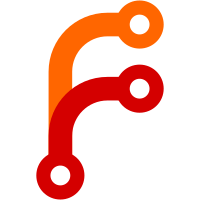
dce8c4c381
rpc: getblockfrompeer (Sjors Provoost)b884ababc2
rpc: move Ensure* helpers to server_util.h (Sjors Provoost) Pull request description: This adds an RPC method to fetch a block directly from a peer. This can used to fetch stale blocks with lower proof of work that are normally ignored by the node (`headers-only` in `getchaintips`). Usage: ``` bitcoin-cli getblockfrompeer HASH peer_n ``` Closes #20155 Limitations: * you have to specify which peer to fetch the block from * the node must already have the header ACKs for top commit: jnewbery: ACKdce8c4c381
fjahr: re-ACKdce8c4c381
Tree-SHA512: 843ba2b7a308f640770d624d0aa3265fdc5c6ea48e8db32269b96a082b7420f7953d1d8d1ef2e6529392c7172dded9d15639fbc9c24e7bfa5cfb79e13a5498c8
67 lines
2 KiB
C++
67 lines
2 KiB
C++
// Copyright (c) 2017-2020 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_RPC_BLOCKCHAIN_H
|
|
#define BITCOIN_RPC_BLOCKCHAIN_H
|
|
|
|
#include <consensus/amount.h>
|
|
#include <core_io.h>
|
|
#include <fs.h>
|
|
#include <streams.h>
|
|
#include <sync.h>
|
|
|
|
#include <any>
|
|
#include <stdint.h>
|
|
#include <vector>
|
|
|
|
extern RecursiveMutex cs_main;
|
|
|
|
class CBlock;
|
|
class CBlockIndex;
|
|
class CChainState;
|
|
class CTxMemPool;
|
|
class ChainstateManager;
|
|
class UniValue;
|
|
struct NodeContext;
|
|
|
|
static constexpr int NUM_GETBLOCKSTATS_PERCENTILES = 5;
|
|
|
|
/**
|
|
* Get the difficulty of the net wrt to the given block index.
|
|
*
|
|
* @return A floating point number that is a multiple of the main net minimum
|
|
* difficulty (4295032833 hashes).
|
|
*/
|
|
double GetDifficulty(const CBlockIndex* blockindex);
|
|
|
|
/** Callback for when block tip changed. */
|
|
void RPCNotifyBlockChange(const CBlockIndex*);
|
|
|
|
/** Block description to JSON */
|
|
UniValue blockToJSON(const CBlock& block, const CBlockIndex* tip, const CBlockIndex* blockindex, TxVerbosity verbosity) LOCKS_EXCLUDED(cs_main);
|
|
|
|
/** Mempool information to JSON */
|
|
UniValue MempoolInfoToJSON(const CTxMemPool& pool);
|
|
|
|
/** Mempool to JSON */
|
|
UniValue MempoolToJSON(const CTxMemPool& pool, bool verbose = false, bool include_mempool_sequence = false);
|
|
|
|
/** Block header to JSON */
|
|
UniValue blockheaderToJSON(const CBlockIndex* tip, const CBlockIndex* blockindex) LOCKS_EXCLUDED(cs_main);
|
|
|
|
/** Used by getblockstats to get feerates at different percentiles by weight */
|
|
void CalculatePercentilesByWeight(CAmount result[NUM_GETBLOCKSTATS_PERCENTILES], std::vector<std::pair<CAmount, int64_t>>& scores, int64_t total_weight);
|
|
|
|
/**
|
|
* Helper to create UTXO snapshots given a chainstate and a file handle.
|
|
* @return a UniValue map containing metadata about the snapshot.
|
|
*/
|
|
UniValue CreateUTXOSnapshot(
|
|
NodeContext& node,
|
|
CChainState& chainstate,
|
|
CAutoFile& afile,
|
|
const fs::path& path,
|
|
const fs::path& tmppath);
|
|
|
|
#endif // BITCOIN_RPC_BLOCKCHAIN_H
|