mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-05 10:17:30 -05:00
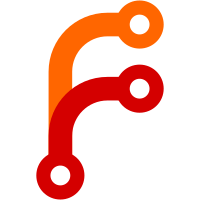
4f7127d1e3
gui: Make Intro consistent with prune checkbox (Hennadii Stepanov)4824a7d36c
gui: Add Intro::UpdateFreeSpaceLabel() (Hennadii Stepanov)daa3f3fa90
refactor: Add Intro::UpdatePruneLabels() (Hennadii Stepanov)e4caa82a03
refactor: Replace static variable with data member (Hennadii Stepanov)2bede28cd9
util: Add PruneGBtoMiB() function (Hennadii Stepanov)e35e4b2ba0
util: Add PruneMiBtoGB() function (Hennadii Stepanov) Pull request description: On master (a6f6333ba2
) and on 0.19.0.1 the intro dialog with prune enabled (checkbox "Discard blocks..." is checked) provides a user with wrong info about the required disk space: 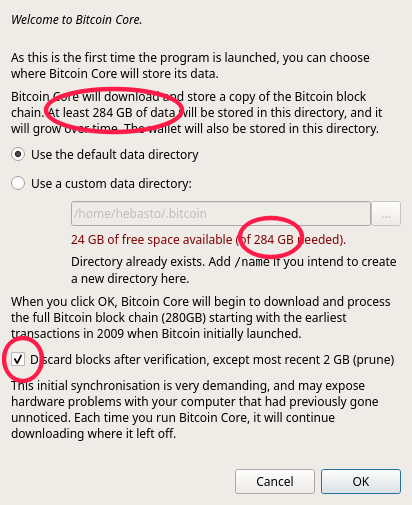 Also the paragraph "If you have chosen to limit..." is missed. --- With this PR when prune checkbox is toggled, the related text labels and the amount of required space shown are updated (previously they were only updated when the data directory was updated): 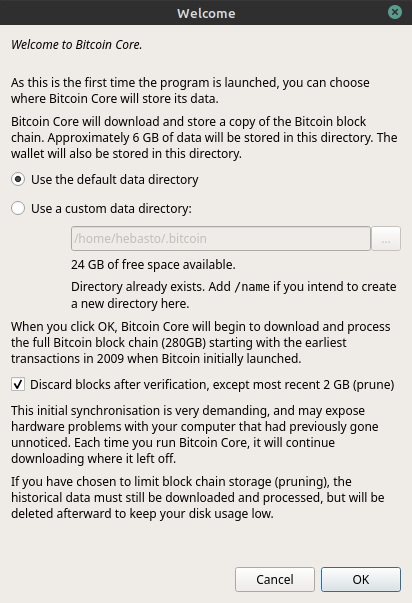 --- This PR is an alternative to #17035. **ryanofsky**'s [suggestion](https://github.com/bitcoin/bitcoin/pull/17035#discussion_r337594268) also has been implemented. ACKs for top commit: emilengler: ACK4f7127d1e3
Sjors: tACK4f7127d1e3
ryanofsky: Code review ACK4f7127d1e3
. It seems like there are a few visible changes here: jonasschnelli: utACK4f7127d1e3
Tree-SHA512: fa0bbdcfafde97d7906cda066cbd4608b936a71cae1b4cda3ee3aa2eed3a9795f279f14c6b1b4997278e094db891c7d3bb695368ba0882347aa42165a86e5172
120 lines
4.2 KiB
C++
120 lines
4.2 KiB
C++
// Copyright (c) 2011-2020 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_QT_OPTIONSMODEL_H
|
|
#define BITCOIN_QT_OPTIONSMODEL_H
|
|
|
|
#include <amount.h>
|
|
#include <qt/guiconstants.h>
|
|
|
|
#include <QAbstractListModel>
|
|
|
|
namespace interfaces {
|
|
class Node;
|
|
}
|
|
|
|
extern const char *DEFAULT_GUI_PROXY_HOST;
|
|
static constexpr unsigned short DEFAULT_GUI_PROXY_PORT = 9050;
|
|
|
|
/**
|
|
* Convert configured prune target MiB to displayed GB. Round up to avoid underestimating max disk usage.
|
|
*/
|
|
static inline int PruneMiBtoGB(int64_t mib) { return (mib * 1024 * 1024 + GB_BYTES - 1) / GB_BYTES; }
|
|
|
|
/**
|
|
* Convert displayed prune target GB to configured MiB. Round down so roundtrip GB -> MiB -> GB conversion is stable.
|
|
*/
|
|
static inline int64_t PruneGBtoMiB(int gb) { return gb * GB_BYTES / 1024 / 1024; }
|
|
|
|
/** Interface from Qt to configuration data structure for Bitcoin client.
|
|
To Qt, the options are presented as a list with the different options
|
|
laid out vertically.
|
|
This can be changed to a tree once the settings become sufficiently
|
|
complex.
|
|
*/
|
|
class OptionsModel : public QAbstractListModel
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
explicit OptionsModel(interfaces::Node& node, QObject *parent = nullptr, bool resetSettings = false);
|
|
|
|
enum OptionID {
|
|
StartAtStartup, // bool
|
|
HideTrayIcon, // bool
|
|
MinimizeToTray, // bool
|
|
MapPortUPnP, // bool
|
|
MinimizeOnClose, // bool
|
|
ProxyUse, // bool
|
|
ProxyIP, // QString
|
|
ProxyPort, // int
|
|
ProxyUseTor, // bool
|
|
ProxyIPTor, // QString
|
|
ProxyPortTor, // int
|
|
DisplayUnit, // BitcoinUnits::Unit
|
|
ThirdPartyTxUrls, // QString
|
|
Language, // QString
|
|
CoinControlFeatures, // bool
|
|
ThreadsScriptVerif, // int
|
|
Prune, // bool
|
|
PruneSize, // int
|
|
DatabaseCache, // int
|
|
SpendZeroConfChange, // bool
|
|
Listen, // bool
|
|
OptionIDRowCount,
|
|
};
|
|
|
|
void Init(bool resetSettings = false);
|
|
void Reset();
|
|
|
|
int rowCount(const QModelIndex & parent = QModelIndex()) const;
|
|
QVariant data(const QModelIndex & index, int role = Qt::DisplayRole) const;
|
|
bool setData(const QModelIndex & index, const QVariant & value, int role = Qt::EditRole);
|
|
/** Updates current unit in memory, settings and emits displayUnitChanged(newUnit) signal */
|
|
void setDisplayUnit(const QVariant &value);
|
|
|
|
/* Explicit getters */
|
|
bool getHideTrayIcon() const { return fHideTrayIcon; }
|
|
bool getMinimizeToTray() const { return fMinimizeToTray; }
|
|
bool getMinimizeOnClose() const { return fMinimizeOnClose; }
|
|
int getDisplayUnit() const { return nDisplayUnit; }
|
|
QString getThirdPartyTxUrls() const { return strThirdPartyTxUrls; }
|
|
bool getCoinControlFeatures() const { return fCoinControlFeatures; }
|
|
const QString& getOverriddenByCommandLine() { return strOverriddenByCommandLine; }
|
|
|
|
/* Explicit setters */
|
|
void SetPruneEnabled(bool prune, bool force = false);
|
|
void SetPruneTargetGB(int prune_target_gb, bool force = false);
|
|
|
|
/* Restart flag helper */
|
|
void setRestartRequired(bool fRequired);
|
|
bool isRestartRequired() const;
|
|
|
|
interfaces::Node& node() const { return m_node; }
|
|
|
|
private:
|
|
interfaces::Node& m_node;
|
|
/* Qt-only settings */
|
|
bool fHideTrayIcon;
|
|
bool fMinimizeToTray;
|
|
bool fMinimizeOnClose;
|
|
QString language;
|
|
int nDisplayUnit;
|
|
QString strThirdPartyTxUrls;
|
|
bool fCoinControlFeatures;
|
|
/* settings that were overridden by command-line */
|
|
QString strOverriddenByCommandLine;
|
|
|
|
// Add option to list of GUI options overridden through command line/config file
|
|
void addOverriddenOption(const std::string &option);
|
|
|
|
// Check settings version and upgrade default values if required
|
|
void checkAndMigrate();
|
|
Q_SIGNALS:
|
|
void displayUnitChanged(int unit);
|
|
void coinControlFeaturesChanged(bool);
|
|
void hideTrayIconChanged(bool);
|
|
};
|
|
|
|
#endif // BITCOIN_QT_OPTIONSMODEL_H
|