mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-04 10:07:27 -05:00
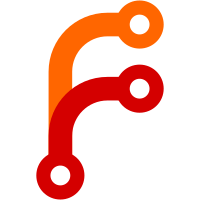
2022917223
Add secp256k1_selftest call (Pieter Wuille)3bfca788b0
Remove explicit enabling of default modules (Pieter Wuille)4462cb0498
Adapt to libsecp256k1 API changes (Pieter Wuille)9d47e7b71b
Squashed 'src/secp256k1/' changes from 44c2452fd3..21ffe4b22a (Pieter Wuille) Pull request description: Now that libsecp256k1 has a release (https://lists.linuxfoundation.org/pipermail/bitcoin-dev/2022-December/021271.html), update the subtree to match it. The changes themselves are not very impactful for Bitcoin Core, but include: * It's no longer needed to specify whether contexts are for signing or verification or both (all contexts support everything), so make use of that in this PR. * Verification operations can use the static context now, removing the need for some infrastructure in pubkey.cpp to make sure a context exists. * Most modules are now enabled by default, so we can drop explicit enabling for them. * CI improvements (in particular, MSVC and more recent MacOS) * Introduction of an internal int128 type, which has no effect for GCC/Clang builds, but enables 128-bit multiplication in MSVC, giving a ~20% speedup there (but still slower than GCC/Clang). * Release process changes (process documentation, changelog, ...). ACKs for top commit: Sjors: ACK2022917223
, but4462cb0498
could use more eyes on it. achow101: ACK2022917223
jonasnick: utACK2022917223
Tree-SHA512: 8a9fe28852abe74abd6f96fef16a94d5a427b1d99bff4caab1699014d24698aab9b966a5364a46ed1001c07a7c1d825154ed4e6557c7decce952b77330a8616b
53 lines
2 KiB
C++
53 lines
2 KiB
C++
// Copyright (c) 2016-2022 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <bench/bench.h>
|
|
#include <coins.h>
|
|
#include <policy/policy.h>
|
|
#include <script/signingprovider.h>
|
|
#include <test/util/transaction_utils.h>
|
|
|
|
#include <vector>
|
|
|
|
// Microbenchmark for simple accesses to a CCoinsViewCache database. Note from
|
|
// laanwj, "replicating the actual usage patterns of the client is hard though,
|
|
// many times micro-benchmarks of the database showed completely different
|
|
// characteristics than e.g. reindex timings. But that's not a requirement of
|
|
// every benchmark."
|
|
// (https://github.com/bitcoin/bitcoin/issues/7883#issuecomment-224807484)
|
|
static void CCoinsCaching(benchmark::Bench& bench)
|
|
{
|
|
ECC_Start();
|
|
|
|
FillableSigningProvider keystore;
|
|
CCoinsView coinsDummy;
|
|
CCoinsViewCache coins(&coinsDummy);
|
|
std::vector<CMutableTransaction> dummyTransactions =
|
|
SetupDummyInputs(keystore, coins, {11 * COIN, 50 * COIN, 21 * COIN, 22 * COIN});
|
|
|
|
CMutableTransaction t1;
|
|
t1.vin.resize(3);
|
|
t1.vin[0].prevout.hash = dummyTransactions[0].GetHash();
|
|
t1.vin[0].prevout.n = 1;
|
|
t1.vin[0].scriptSig << std::vector<unsigned char>(65, 0);
|
|
t1.vin[1].prevout.hash = dummyTransactions[1].GetHash();
|
|
t1.vin[1].prevout.n = 0;
|
|
t1.vin[1].scriptSig << std::vector<unsigned char>(65, 0) << std::vector<unsigned char>(33, 4);
|
|
t1.vin[2].prevout.hash = dummyTransactions[1].GetHash();
|
|
t1.vin[2].prevout.n = 1;
|
|
t1.vin[2].scriptSig << std::vector<unsigned char>(65, 0) << std::vector<unsigned char>(33, 4);
|
|
t1.vout.resize(2);
|
|
t1.vout[0].nValue = 90 * COIN;
|
|
t1.vout[0].scriptPubKey << OP_1;
|
|
|
|
// Benchmark.
|
|
const CTransaction tx_1(t1);
|
|
bench.run([&] {
|
|
bool success{AreInputsStandard(tx_1, coins)};
|
|
assert(success);
|
|
});
|
|
ECC_Stop();
|
|
}
|
|
|
|
BENCHMARK(CCoinsCaching, benchmark::PriorityLevel::HIGH);
|