mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-04 10:07:27 -05:00
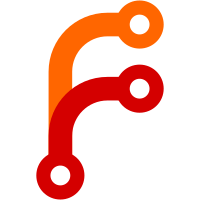
aeb18b665c
Bugfix: GUI: Check validity when QValidatedLineEdit::setText is called (Luke Dashjr)b1a544be10
Bugfix: GUI: Re-check validity after QValidatedLineEdit::setCheckValidator (Luke Dashjr)2385b508d5
Bugfix: GUI: Only apply invalid style to QValidatedLineEdit, not its tooltip (Luke Dashjr) Pull request description: 1. Use a CSS selector to avoid changing the background colour of the tooltip. 2. Re-check validity of input when we first set the validator (probably a no-op in practice). 3. Check validity of input when it is set programmatically via `setText` (eg, via the address book). Probably no-op in practice UNTIL merging https://github.com/bitcoin/bitcoin/pull/15987 or any other PR that adds a warning for valid addresses. Moved from https://github.com/bitcoin/bitcoin/pull/18133 (just concept ACKs) ACKs for top commit: Sjors: tACKaeb18b665c
hebasto: ACKaeb18b665c
, tested on Linux Mint 20.3 (Qt 5.12.8). Tree-SHA512: b6fa8ee4dec76e1c759095721240e6fa5071a02993cb28406e96a0fa2e819f5dddc03d2e7c9073354d7865c2b09eb263afaf853ecba42e9fc4f50ef4ae20bf0f
130 lines
2.7 KiB
C++
130 lines
2.7 KiB
C++
// Copyright (c) 2011-2018 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <qt/qvalidatedlineedit.h>
|
|
|
|
#include <qt/bitcoinaddressvalidator.h>
|
|
#include <qt/guiconstants.h>
|
|
|
|
QValidatedLineEdit::QValidatedLineEdit(QWidget *parent) :
|
|
QLineEdit(parent),
|
|
valid(true),
|
|
checkValidator(nullptr)
|
|
{
|
|
connect(this, &QValidatedLineEdit::textChanged, this, &QValidatedLineEdit::markValid);
|
|
}
|
|
|
|
void QValidatedLineEdit::setText(const QString& text)
|
|
{
|
|
QLineEdit::setText(text);
|
|
checkValidity();
|
|
}
|
|
|
|
void QValidatedLineEdit::setValid(bool _valid)
|
|
{
|
|
if(_valid == this->valid)
|
|
{
|
|
return;
|
|
}
|
|
|
|
if(_valid)
|
|
{
|
|
setStyleSheet("");
|
|
}
|
|
else
|
|
{
|
|
setStyleSheet("QValidatedLineEdit { " STYLE_INVALID "}");
|
|
}
|
|
this->valid = _valid;
|
|
}
|
|
|
|
void QValidatedLineEdit::focusInEvent(QFocusEvent *evt)
|
|
{
|
|
// Clear invalid flag on focus
|
|
setValid(true);
|
|
|
|
QLineEdit::focusInEvent(evt);
|
|
}
|
|
|
|
void QValidatedLineEdit::focusOutEvent(QFocusEvent *evt)
|
|
{
|
|
checkValidity();
|
|
|
|
QLineEdit::focusOutEvent(evt);
|
|
}
|
|
|
|
void QValidatedLineEdit::markValid()
|
|
{
|
|
// As long as a user is typing ensure we display state as valid
|
|
setValid(true);
|
|
}
|
|
|
|
void QValidatedLineEdit::clear()
|
|
{
|
|
setValid(true);
|
|
QLineEdit::clear();
|
|
}
|
|
|
|
void QValidatedLineEdit::setEnabled(bool enabled)
|
|
{
|
|
if (!enabled)
|
|
{
|
|
// A disabled QValidatedLineEdit should be marked valid
|
|
setValid(true);
|
|
}
|
|
else
|
|
{
|
|
// Recheck validity when QValidatedLineEdit gets enabled
|
|
checkValidity();
|
|
}
|
|
|
|
QLineEdit::setEnabled(enabled);
|
|
}
|
|
|
|
void QValidatedLineEdit::checkValidity()
|
|
{
|
|
if (text().isEmpty())
|
|
{
|
|
setValid(true);
|
|
}
|
|
else if (hasAcceptableInput())
|
|
{
|
|
setValid(true);
|
|
|
|
// Check contents on focus out
|
|
if (checkValidator)
|
|
{
|
|
QString address = text();
|
|
int pos = 0;
|
|
if (checkValidator->validate(address, pos) == QValidator::Acceptable)
|
|
setValid(true);
|
|
else
|
|
setValid(false);
|
|
}
|
|
}
|
|
else
|
|
setValid(false);
|
|
|
|
Q_EMIT validationDidChange(this);
|
|
}
|
|
|
|
void QValidatedLineEdit::setCheckValidator(const QValidator *v)
|
|
{
|
|
checkValidator = v;
|
|
checkValidity();
|
|
}
|
|
|
|
bool QValidatedLineEdit::isValid()
|
|
{
|
|
// use checkValidator in case the QValidatedLineEdit is disabled
|
|
if (checkValidator)
|
|
{
|
|
QString address = text();
|
|
int pos = 0;
|
|
if (checkValidator->validate(address, pos) == QValidator::Acceptable)
|
|
return true;
|
|
}
|
|
|
|
return valid;
|
|
}
|