mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-04 10:07:27 -05:00
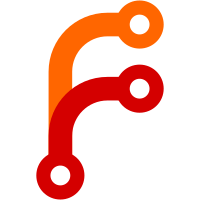
This is a minimal extraction of a single function, but also the only use of std::exception in util/system. The background of this commit is an ongoing effort to decouple the libbitcoinkernel library from the ArgsManager defined in system.h. Moving the function out of system.h allows including it from a separate source file without including the ArgsManager definitions from system.h.
41 lines
1.2 KiB
C++
41 lines
1.2 KiB
C++
// Copyright (c) 2009-2010 Satoshi Nakamoto
|
|
// Copyright (c) 2009-2023 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <util/exception.h>
|
|
|
|
#include <logging.h>
|
|
#include <tinyformat.h>
|
|
|
|
#include <exception>
|
|
#include <iostream>
|
|
#include <string>
|
|
#include <typeinfo>
|
|
|
|
#ifdef WIN32
|
|
#include <windows.h>
|
|
#endif // WIN32
|
|
|
|
static std::string FormatException(const std::exception* pex, std::string_view thread_name)
|
|
{
|
|
#ifdef WIN32
|
|
char pszModule[MAX_PATH] = "";
|
|
GetModuleFileNameA(nullptr, pszModule, sizeof(pszModule));
|
|
#else
|
|
const char* pszModule = "bitcoin";
|
|
#endif
|
|
if (pex)
|
|
return strprintf(
|
|
"EXCEPTION: %s \n%s \n%s in %s \n", typeid(*pex).name(), pex->what(), pszModule, thread_name);
|
|
else
|
|
return strprintf(
|
|
"UNKNOWN EXCEPTION \n%s in %s \n", pszModule, thread_name);
|
|
}
|
|
|
|
void PrintExceptionContinue(const std::exception* pex, std::string_view thread_name)
|
|
{
|
|
std::string message = FormatException(pex, thread_name);
|
|
LogPrintf("\n\n************************\n%s\n", message);
|
|
tfm::format(std::cerr, "\n\n************************\n%s\n", message);
|
|
}
|