mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-02-04 10:07:27 -05:00
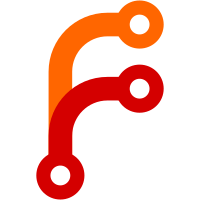
ecc6cf1a3b
test: fix creation of std::string objects with \0s (Vasil Dimov) Pull request description: A string literal `"abc"` contains a terminating `\0`, so that is 4 bytes. There is no need to write `"abc\0"` unless two terminating `\0`s are necessary. `std::string` objects do not internally contain a terminating `\0`, so `std::string("abc")` creates a string with size 3 and is the same as `std::string("abc", 3)`. In `"\01"` the `01` part is interpreted as one number (1) and that is the same as `"\1"` which is a string like `{1, 0}` whereas `"\0z"` is a string like `{0, 'z', 0}`. To create a string like `{0, '1', 0}` one must use `"\0" "1"`. Adjust the tests accordingly. ACKs for top commit: laanwj: ACKecc6cf1a3b
practicalswift: ACKecc6cf1a3b
modulo happily green CI Tree-SHA512: 5eb489e8533a4199a9324b92f7280041552379731ebf7dfee169f70d5458e20e29b36f8bfaee6f201f48ab2b9d1d0fc4bdf8d6e4c58d6102f399cfbea54a219e
42 lines
1.6 KiB
C++
42 lines
1.6 KiB
C++
// Copyright (c) 2012-2020 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include <test/util/setup_common.h>
|
|
#include <util/strencodings.h>
|
|
|
|
#include <boost/test/unit_test.hpp>
|
|
#include <string>
|
|
|
|
using namespace std::literals;
|
|
|
|
BOOST_FIXTURE_TEST_SUITE(base32_tests, BasicTestingSetup)
|
|
|
|
BOOST_AUTO_TEST_CASE(base32_testvectors)
|
|
{
|
|
static const std::string vstrIn[] = {"","f","fo","foo","foob","fooba","foobar"};
|
|
static const std::string vstrOut[] = {"","my======","mzxq====","mzxw6===","mzxw6yq=","mzxw6ytb","mzxw6ytboi======"};
|
|
static const std::string vstrOutNoPadding[] = {"","my","mzxq","mzxw6","mzxw6yq","mzxw6ytb","mzxw6ytboi"};
|
|
for (unsigned int i=0; i<sizeof(vstrIn)/sizeof(vstrIn[0]); i++)
|
|
{
|
|
std::string strEnc = EncodeBase32(vstrIn[i]);
|
|
BOOST_CHECK_EQUAL(strEnc, vstrOut[i]);
|
|
strEnc = EncodeBase32(vstrIn[i], false);
|
|
BOOST_CHECK_EQUAL(strEnc, vstrOutNoPadding[i]);
|
|
std::string strDec = DecodeBase32(vstrOut[i]);
|
|
BOOST_CHECK_EQUAL(strDec, vstrIn[i]);
|
|
}
|
|
|
|
// Decoding strings with embedded NUL characters should fail
|
|
bool failure;
|
|
(void)DecodeBase32("invalid\0"s, &failure); // correct size, invalid due to \0
|
|
BOOST_CHECK(failure);
|
|
(void)DecodeBase32("AWSX3VPP"s, &failure); // valid
|
|
BOOST_CHECK(!failure);
|
|
(void)DecodeBase32("AWSX3VPP\0invalid"s, &failure); // correct size, invalid due to \0
|
|
BOOST_CHECK(failure);
|
|
(void)DecodeBase32("AWSX3VPPinvalid"s, &failure); // invalid size
|
|
BOOST_CHECK(failure);
|
|
}
|
|
|
|
BOOST_AUTO_TEST_SUITE_END()
|