mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-03-09 15:37:00 -04:00
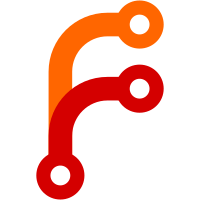
Re-submitting this pull request with a single commit. This patch introduces a GUIUtil class that is used when setting up the 2 tables we have so far on the Qt-GUI. In the past you could only resize the last column, which has BTC amounts from the right border of the column header, something that was rather unnatural. If a new table were ever to be added to the interface, fixing the last columns resizing behavior is rather simple. Just look at how we initialize here a TableViewLastColumnResizingFixer object when setting up the table header's behavior, and then how we override the resize event of the component (can be the table, or the dialog) and we invoke columnResizingFixer->stretchColumnWidth(columnIndex);
74 lines
1.8 KiB
C++
74 lines
1.8 KiB
C++
// Copyright (c) 2011-2014 The Bitcoin developers
|
|
// Distributed under the MIT/X11 software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef RECEIVECOINSDIALOG_H
|
|
#define RECEIVECOINSDIALOG_H
|
|
|
|
#include <QDialog>
|
|
#include <QKeyEvent>
|
|
#include <QMenu>
|
|
#include <QPoint>
|
|
#include <QVariant>
|
|
#include <QHeaderView>
|
|
#include <QItemSelection>
|
|
#include "guiutil.h"
|
|
|
|
namespace Ui {
|
|
class ReceiveCoinsDialog;
|
|
}
|
|
class WalletModel;
|
|
class OptionsModel;
|
|
|
|
QT_BEGIN_NAMESPACE
|
|
class QModelIndex;
|
|
QT_END_NAMESPACE
|
|
|
|
/** Dialog for requesting payment of bitcoins */
|
|
class ReceiveCoinsDialog : public QDialog
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
enum ColumnWidths {
|
|
DATE_COLUMN_WIDTH = 130,
|
|
LABEL_COLUMN_WIDTH = 120,
|
|
AMOUNT_MINIMUM_COLUMN_WIDTH = 160,
|
|
MINIMUM_COLUMN_WIDTH = 130
|
|
};
|
|
|
|
explicit ReceiveCoinsDialog(QWidget *parent = 0);
|
|
~ReceiveCoinsDialog();
|
|
void setModel(WalletModel *model);
|
|
|
|
|
|
public slots:
|
|
void clear();
|
|
void reject();
|
|
void accept();
|
|
|
|
protected:
|
|
virtual void keyPressEvent(QKeyEvent *event);
|
|
|
|
private:
|
|
Ui::ReceiveCoinsDialog *ui;
|
|
GUIUtil::TableViewLastColumnResizingFixer *columnResizingFixer;
|
|
WalletModel *model;
|
|
QMenu *contextMenu;
|
|
void copyColumnToClipboard(int column);
|
|
virtual void resizeEvent(QResizeEvent* event);
|
|
|
|
private slots:
|
|
void on_receiveButton_clicked();
|
|
void on_showRequestButton_clicked();
|
|
void on_removeRequestButton_clicked();
|
|
void on_recentRequestsView_doubleClicked(const QModelIndex &index);
|
|
void on_recentRequestsView_selectionChanged(const QItemSelection &, const QItemSelection &);
|
|
void updateDisplayUnit();
|
|
void showMenu(const QPoint &);
|
|
void copyLabel();
|
|
void copyMessage();
|
|
void copyAmount();
|
|
};
|
|
|
|
#endif // RECEIVECOINSDIALOG_H
|