mirror of
https://github.com/denoland/deno.git
synced 2025-02-01 12:16:11 -05:00
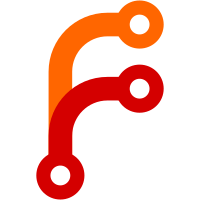
Allows easily constructing a `DenoResolver` using the exact same logic that we use in the CLI (useful for dnt and for external bundlers). This code is then used in the CLI to ensure the logic is always up-to-date. ```rs use std::rc::Rc; use deno_resolver:🏭:ResolverFactory; use deno_resolver:🏭:WorkspaceFactory; use sys_traits::impls::RealSys; let sys = RealSys; let cwd = sys.env_current_dir()?; let workspace_factory = Rc::new(WorkspaceFactory::new(sys, cwd, Default::default())); let resolver_factory = ResolverFactory::new(workspace_factory.clone(), Default::default()); let deno_resolver = resolver_factory.deno_resolver().await?; ```
103 lines
2.1 KiB
Rust
103 lines
2.1 KiB
Rust
// Copyright 2018-2025 the Deno authors. MIT license.
|
|
|
|
pub trait IsBuiltInNodeModuleChecker: std::fmt::Debug {
|
|
/// e.g. `is_builtin_node_module("assert")`
|
|
fn is_builtin_node_module(&self, module_name: &str) -> bool;
|
|
}
|
|
|
|
/// An implementation of IsBuiltInNodeModuleChecker that uses
|
|
/// the list of built-in node_modules that are supported by Deno
|
|
/// in the `deno_node` crate (ext/node).
|
|
#[derive(Debug)]
|
|
pub struct DenoIsBuiltInNodeModuleChecker;
|
|
|
|
impl IsBuiltInNodeModuleChecker for DenoIsBuiltInNodeModuleChecker {
|
|
#[inline(always)]
|
|
fn is_builtin_node_module(&self, module_name: &str) -> bool {
|
|
DENO_SUPPORTED_BUILTIN_NODE_MODULES
|
|
.binary_search(&module_name)
|
|
.is_ok()
|
|
}
|
|
}
|
|
|
|
/// Collection of built-in node_modules supported by Deno.
|
|
pub static DENO_SUPPORTED_BUILTIN_NODE_MODULES: &[&str] = &[
|
|
// NOTE(bartlomieju): keep this list in sync with `ext/node/polyfills/01_require.js`
|
|
"_http_agent",
|
|
"_http_common",
|
|
"_http_outgoing",
|
|
"_http_server",
|
|
"_stream_duplex",
|
|
"_stream_passthrough",
|
|
"_stream_readable",
|
|
"_stream_transform",
|
|
"_stream_writable",
|
|
"_tls_common",
|
|
"_tls_wrap",
|
|
"assert",
|
|
"assert/strict",
|
|
"async_hooks",
|
|
"buffer",
|
|
"child_process",
|
|
"cluster",
|
|
"console",
|
|
"constants",
|
|
"crypto",
|
|
"dgram",
|
|
"diagnostics_channel",
|
|
"dns",
|
|
"dns/promises",
|
|
"domain",
|
|
"events",
|
|
"fs",
|
|
"fs/promises",
|
|
"http",
|
|
"http2",
|
|
"https",
|
|
"inspector",
|
|
"inspector/promises",
|
|
"module",
|
|
"net",
|
|
"os",
|
|
"path",
|
|
"path/posix",
|
|
"path/win32",
|
|
"perf_hooks",
|
|
"process",
|
|
"punycode",
|
|
"querystring",
|
|
"readline",
|
|
"readline/promises",
|
|
"repl",
|
|
"stream",
|
|
"stream/consumers",
|
|
"stream/promises",
|
|
"stream/web",
|
|
"string_decoder",
|
|
"sys",
|
|
"test",
|
|
"timers",
|
|
"timers/promises",
|
|
"tls",
|
|
"tty",
|
|
"url",
|
|
"util",
|
|
"util/types",
|
|
"v8",
|
|
"vm",
|
|
"wasi",
|
|
"worker_threads",
|
|
"zlib",
|
|
];
|
|
|
|
#[cfg(test)]
|
|
mod test {
|
|
use super::*;
|
|
|
|
#[test]
|
|
fn test_builtins_are_sorted() {
|
|
let mut builtins_list = DENO_SUPPORTED_BUILTIN_NODE_MODULES.to_vec();
|
|
builtins_list.sort();
|
|
assert_eq!(DENO_SUPPORTED_BUILTIN_NODE_MODULES, builtins_list);
|
|
}
|
|
}
|